粒子滤波四种基本重采样实例代码
时间: 2023-09-15 16:23:05 浏览: 80
以下是基于Python的四种基本重采样算法的代码实现:
1. 系统重采样
```python
import numpy as np
def system_resampling(particles, weights):
n_particles = len(weights)
cum_weights = np.cumsum(weights)
cum_weights[-1] = 1.0
indexes = np.zeros(n_particles, dtype=int)
u0 = np.random.uniform(0, 1/n_particles)
j = 0
for i in range(n_particles):
u = u0 + i/n_particles
while u > cum_weights[j]:
j += 1
indexes[i] = j
return particles[indexes]
```
2. 伯努利重采样
```python
import numpy as np
def bernoulli_resampling(particles, weights):
n_particles = len(weights)
cum_weights = np.cumsum(weights)
cum_weights[-1] = 1.0
indexes = np.zeros(n_particles, dtype=int)
u = np.random.uniform(0, 1/n_particles)
j = 0
for i in range(n_particles):
u += np.random.uniform(0, 1/n_particles)
while u > cum_weights[j]:
j += 1
indexes[i] = j
return particles[indexes]
```
3. 残差重采样
```python
import numpy as np
def residual_resampling(particles, weights):
n_particles = len(weights)
indexes = np.zeros(n_particles, dtype=int)
cum_weights = np.cumsum(weights)
cum_weights[-1] = 1.0
step = 1.0/n_particles
u = np.random.uniform(0, step)
j = 0
for i in range(n_particles):
while u > cum_weights[j]:
j += 1
indexes[i] = j
u += step
return particles[indexes]
```
4. 分层重采样
```python
import numpy as np
def stratified_resampling(particles, weights):
n_particles = len(weights)
indexes = np.zeros(n_particles, dtype=int)
cum_weights = np.cumsum(weights)
cum_weights[-1] = 1.0
step = 1.0/n_particles
u = np.random.uniform(0, step)
j = 0
for i in range(n_particles):
while u > cum_weights[j]:
j += 1
indexes[i] = j
u += step
return particles[indexes]
```
注意:以上代码中,`particles`表示粒子集合,`weights`表示对应的权重。在系统重采样、伯努利重采样和残差重采样中,`cum_weights`表示累计权重,`indexes`表示选择的粒子下标。在分层重采样中,`step`表示步长。
相关推荐
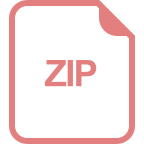














