用c++写一个小飞机大战游戏
时间: 2024-04-05 19:14:07 浏览: 86
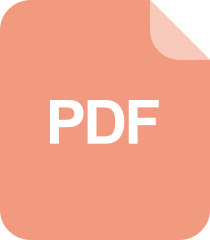
C++编写简易的飞机大战

好的,我可以为您提供一个简单的控制台小飞机大战游戏代码。该游戏使用箭头键控制玩家飞机的移动,空格键发射子弹,按下ESC键退出游戏。
```c++
#include <iostream>
#include <conio.h>
#include <windows.h>
#include <vector>
using namespace std;
const int WIDTH = 80; // 游戏窗口的宽度
const int HEIGHT = 30; // 游戏窗口的高度
// 飞机类
class Plane {
public:
Plane(int x, int y, int lives) : x(x), y(y), lives(lives) {}
int getX() const { return x; }
int getY() const { return y; }
int getLives() const { return lives; }
void move(int dx, int dy);
void shoot(vector<Bullet>& bullets);
private:
int x, y; // 飞机的坐标
int lives; // 飞机的生命值
};
// 子弹类
class Bullet {
public:
Bullet(int x, int y) : x(x), y(y) {}
int getX() const { return x; }
int getY() const { return y; }
void move();
private:
int x, y; // 子弹的坐标
};
// 游戏类
class Game {
public:
Game();
void run();
private:
void draw(); // 绘制游戏界面
void update(); // 更新游戏状态
void processInput(); // 处理用户输入
void fireBullet(); // 发射子弹
bool isCollided(const Bullet& bullet, const Plane& plane); // 判断子弹是否撞到飞机
void gameOver(); // 游戏结束
Plane player; // 玩家飞机
vector<Bullet> bullets; // 所有子弹
int score; // 得分
bool isGameOver; // 游戏是否结束
};
// 飞机移动
void Plane::move(int dx, int dy) {
x += dx;
y += dy;
if (x < 0) x = 0;
if (x > WIDTH - 1) x = WIDTH - 1;
if (y < 0) y = 0;
if (y > HEIGHT - 1) y = HEIGHT - 1;
}
// 发射子弹
void Plane::shoot(vector<Bullet>& bullets) {
bullets.push_back(Bullet(x, y - 1));
}
// 子弹移动
void Bullet::move() {
y--;
}
// 游戏初始化
Game::Game() : player(WIDTH / 2, HEIGHT - 2, 3), score(0), isGameOver(false) {
// 隐藏光标
HANDLE hStdOut = GetStdHandle(STD_OUTPUT_HANDLE);
CONSOLE_CURSOR_INFO cci;
GetConsoleCursorInfo(hStdOut, &cci);
cci.bVisible = FALSE;
SetConsoleCursorInfo(hStdOut, &cci);
}
// 绘制游戏界面
void Game::draw() {
system("cls"); // 清空屏幕
// 绘制玩家飞机
for (int i = 0; i < player.getLives(); i++) {
cout << " ^ " << endl;
cout << " / \\ " << endl;
cout << "/ \\" << endl;
}
// 绘制所有子弹
for (auto& bullet : bullets) {
cout << " | " << endl;
cout << " * " << endl;
cout << " | " << endl;
}
// 输出得分
cout << "Score: " << score << endl;
}
// 更新游戏状态
void Game::update() {
// 移动所有子弹
for (auto& bullet : bullets) {
bullet.move();
}
// 检查是否有子弹撞到飞机
for (auto& bullet : bullets) {
if (isCollided(bullet, player)) {
player = Plane(WIDTH / 2, HEIGHT - 2, player.getLives() - 1);
if (player.getLives() == 0) {
gameOver();
return;
}
}
}
// 移除所有已经超出屏幕的子弹
bullets.erase(remove_if(bullets.begin(), bullets.end(), [](const Bullet& bullet) {
return bullet.getY() < 0;
}), bullets.end());
// 更新得分
score++;
// 更新游戏界面
draw();
}
// 处理用户输入
void Game::processInput() {
if (_kbhit()) {
char ch = _getch();
switch (ch) {
case 27: // ESC键
gameOver();
break;
case ' ': // 空格键
fireBullet();
break;
case 'a':
case 'A':
player.move(-1, 0);
break;
case 'd':
case 'D':
player.move(1, 0);
break;
case 'w':
case 'W':
player.move(0, -1);
break;
case 's':
case 'S':
player.move(0, 1);
break;
}
}
}
// 发射子弹
void Game::fireBullet() {
player.shoot(bullets);
}
// 判断子弹是否撞到飞机
bool Game::isCollided(const Bullet& bullet, const Plane& plane) {
int x = bullet.getX();
int y = bullet.getY();
int px = plane.getX();
int py = plane.getY();
int lives = plane.getLives();
return (x >= px - 1 && x <= px + 1 && y == py) || (y == py - 1 && x == px && lives > 0);
}
// 游戏结束
void Game::gameOver() {
isGameOver = true;
system("cls");
cout << "Game Over!" << endl;
cout << "Your score is: " << score << endl;
}
// 运行游戏
void Game::run() {
while (!isGameOver) {
processInput();
update();
Sleep(50);
}
}
int main() {
Game game;
game.run();
return 0;
}
```
这个游戏有一个玩家飞机和多个子弹。玩家可以使用箭头键控制飞机的移动,使用空格键发射子弹。当子弹撞到飞机时,飞机的生命值减少,当生命值为零时游戏结束。游戏会根据玩家的得分不断更新游戏界面。
阅读全文
相关推荐
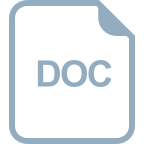
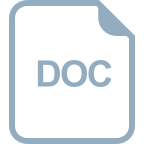



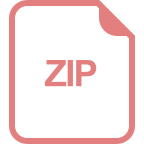
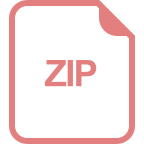
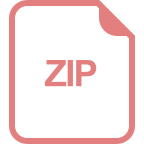
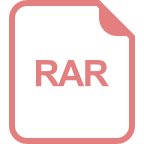
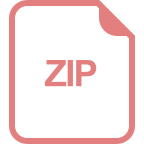
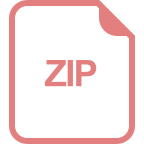
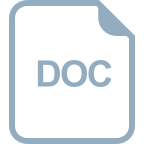
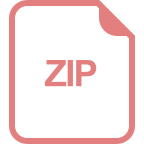



