用c语言编写程序,实现FPF算法,模拟进程调度过程
时间: 2023-12-16 16:02:56 浏览: 328
FPF(First Present Fit)算法是一种内存分配算法,常用于操作系统中的内存管理。它的基本思想是在空闲内存块中选择第一个能够容纳所需内存的块进行分配。
下面是一个使用 C 语言实现 FPF 算法的简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_MEMORY_SIZE 1024
int memory[MAX_MEMORY_SIZE]; // 模拟内存空间
// 内存块结构体
typedef struct block {
int start; // 起始位置
int size; // 大小
int used; // 是否已被使用
} Block;
Block blocks[MAX_MEMORY_SIZE]; // 内存块数组
int blockCount = 0; // 内存块数量
// 初始化内存块
void initBlocks() {
blockCount = 1;
blocks[0].start = 0;
blocks[0].size = MAX_MEMORY_SIZE;
blocks[0].used = 0;
}
// 打印内存块信息
void printBlocks() {
printf("Memory blocks:\n");
for (int i = 0; i < blockCount; i++) {
printf("Block %d: start=%d, size=%d, used=%d\n", i, blocks[i].start, blocks[i].size, blocks[i].used);
}
}
// 分配内存
int allocateMemory(int size) {
for (int i = 0; i < blockCount; i++) {
if (!blocks[i].used && blocks[i].size >= size) { // 找到第一个能够容纳所需内存的块
int start = blocks[i].start;
blocks[i].start += size;
blocks[i].size -= size;
if (blocks[i].size == 0) { // 如果内存块完全使用,则删除该块
for (int j = i; j < blockCount - 1; j++) {
blocks[j] = blocks[j + 1];
}
blockCount--;
} else { // 否则,新建一个内存块
blocks[blockCount].start = start + size;
blocks[blockCount].size = blocks[i].size;
blocks[blockCount].used = 0;
blockCount++;
}
blocks[i].used = 1;
return start;
}
}
return -1; // 内存不足,分配失败
}
// 释放内存
void freeMemory(int start, int size) {
int end = start + size;
for (int i = 0; i < blockCount; i++) {
if (blocks[i].start + blocks[i].size == start && !blocks[i].used) { // 合并相邻的空闲块
blocks[i].size += size;
start = blocks[i].start;
size = blocks[i].size;
for (int j = i; j < blockCount - 1; j++) {
blocks[j] = blocks[j + 1];
}
blockCount--;
break;
} else if (blocks[i].start == end && !blocks[i].used) {
blocks[i].start = start;
blocks[i].size += size;
start = blocks[i].start;
size = blocks[i].size;
for (int j = i; j < blockCount - 1; j++) {
blocks[j] = blocks[j + 1];
}
blockCount--;
break;
}
}
blocks[blockCount].start = start;
blocks[blockCount].size = size;
blocks[blockCount].used = 0;
blockCount++;
}
int main() {
initBlocks();
printBlocks();
int start1 = allocateMemory(100);
printf("Allocate 100 bytes, start at %d\n", start1);
printBlocks();
int start2 = allocateMemory(200);
printf("Allocate 200 bytes, start at %d\n", start2);
printBlocks();
int start3 = allocateMemory(50);
printf("Allocate 50 bytes, start at %d\n", start3);
printBlocks();
freeMemory(start1, 100);
printf("Free 100 bytes from %d\n", start1);
printBlocks();
int start4 = allocateMemory(150);
printf("Allocate 150 bytes, start at %d\n", start4);
printBlocks();
freeMemory(start2, 200);
printf("Free 200 bytes from %d\n", start2);
printBlocks();
int start5 = allocateMemory(300);
printf("Allocate 300 bytes, start at %d\n", start5);
printBlocks();
return 0;
}
```
该程序模拟了一块大小为 1024 字节的内存空间,并实现了 FPF 算法的内存分配与释放。运行程序,可以看到内存块的变化过程和相关信息。
阅读全文
相关推荐










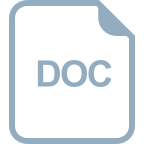


