用resnet18对四种天气分类,自定义归一化方法,采用均值方差归一化方法
时间: 2023-11-04 07:08:44 浏览: 37
好的,以下是使用ResNet18对四种天气进行分类并自定义归一化方法的代码:
```python
import torch
import torch.nn as nn
import torchvision.models as models
# 自定义均值方差归一化方法
class MyNormalize(nn.Module):
def __init__(self, mean, std):
super(MyNormalize, self).__init__()
self.mean = torch.tensor(mean).reshape(1, 3, 1, 1)
self.std = torch.tensor(std).reshape(1, 3, 1, 1)
def forward(self, x):
return (x - self.mean) / self.std
# 加载ResNet18模型
model = models.resnet18(pretrained=True)
# 冻结模型所有参数
for param in model.parameters():
param.requires_grad = False
# 替换最后一层分类器
num_ftrs = model.fc.in_features
model.fc = nn.Linear(num_ftrs, 4)
# 自定义均值方差归一化方法
normalize = MyNormalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.fc.parameters(), lr=0.001, momentum=0.9)
# 训练模型
num_epochs = 10
for epoch in range(num_epochs):
for images, labels in train_loader:
images = normalize(images) # 归一化
outputs = model(images)
loss = criterion(outputs, labels)
optimizer.zero_grad()
loss.backward()
optimizer.step()
# 计算在验证集上的准确率
correct = 0
total = 0
with torch.no_grad():
for images, labels in val_loader:
images = normalize(images) # 归一化
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
accuracy = 100 * correct / total
print('Epoch [{}/{}], Accuracy: {:.2f}%'.format(epoch+1, num_epochs, accuracy))
```
其中,`MyNormalize`是自定义的归一化方法,其输入为一个张量,输出为将每个像素减去均值并除以方差后的结果。在模型训练和验证时,需要先将数据归一化后再输入模型中。
相关推荐
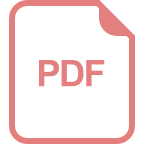
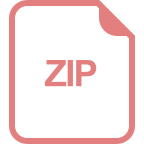
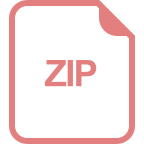









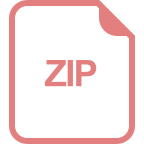
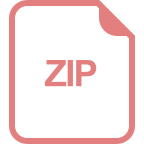
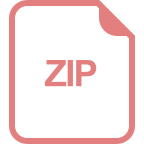
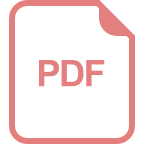