STM32F103C8T6 PORTB时钟
时间: 2023-08-29 11:07:46 浏览: 120
要使用STM32F103C8T6芯片的PORTB,需要先开启PORTB时钟。具体步骤如下:
1. 打开RCC_AHBENR寄存器,使能GPIOB时钟,代码如下:
```
RCC->AHBENR |= RCC_AHBENR_GPIOBEN;
```
2. 配置GPIOB的引脚模式和输出类型,代码如下:
```
GPIOB->CRL &= ~(GPIO_CRL_MODE0 | GPIO_CRL_CNF0);
GPIOB->CRL |= GPIO_CRL_MODE0_1; //推挽输出,最大速率50MHz
```
其中,MODE0_1表示输出模式为推挽输出,最大速率为50MHz。
完成以上两步操作后,就可以使用GPIOB引脚进行输入输出操作了。
相关问题
stm32f103c8t6单片机使用DHT11温湿度监测模块的核心程序
本文给出stm32f103c8t6单片机使用DHT11温湿度监测模块的核心程序,使用C语言开发,可供参考。程序包括DHT11初始化以及读取数据的函数。
1. DHT11初始化
```
void DHT11_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* 使能 PORTB 时钟 */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
/* 配置 PB12 为输出 */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_12;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
/* 设置 PB12 输出高电平 */
GPIO_SetBits(GPIOB, GPIO_Pin_12);
}
```
2. 读取DHT11数据
```
u8 DHT11_Read_Data(u8 *temp,u8 *humi)
{
u8 i=0,j=0;
/* 数组清零操作 */
humi[0]=humi[1]=temp[0]=temp[1]=temp[2]=temp[3]=0;
/* 输出数据总线设置为低电平 */
GPIO_ResetBits(GPIOB, GPIO_Pin_12);
/* 延时18ms */
Delay_ms(18);
/* 输出数据总线设置为高电平 */
GPIO_SetBits(GPIOB, GPIO_Pin_12);
/* 设置数据总线为输入模式 */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_12;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOB, &GPIO_InitStructure);
/* 检测 DHT11 响应信号,判断是否有效 */
if(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_12)!=Bit_RESET)
{
/* 数据总线未检测到有效响应,返回错误 */
return DHT11_ERROR_RESPONSE;
}
/* DHT11 数据位,从高到低依次读取 5Bytes 数据,每个字节 8bit */
for(i=0;i<5;i++)
{
for(j=0;j<8;j++)
{
/* 接收数据,时间片为 45us,最高电平持续时间大于等于 70us 表示高电平,否则为低电平 */
while(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_12)==Bit_RESET); /* 等待数据总线变高 */
Delay_us(50);
if(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_12)==Bit_SET)
{
/* 高电平,数据位为 1 */
humi[i%2]|=(0x80>>j); /* 数据位依次存入 humi 数组 */
}
while(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_12)==Bit_SET); /* 等待数据总线变低 */
}
}
/* 重置数据总线 */
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_SetBits(GPIOB, GPIO_Pin_12);
/* 校验读取数据是否正确 */
if(humi[0]+humi[1]+temp[0]+temp[1]+temp[2]+temp[3]!=humi[2])
{
/* 数据校验出错,返回错误 */
return DHT11_ERROR_CHECKSUM;
}
return DHT11_SUCCESS;
}
```
这段代码将气温数据存储在temp变量中,湿度数据存储在humi变量中,并且验证数据的正确性。同时,需要注意的是,这里使用了delay函数,程序中需要定义delay_ms和delay_us两个函数,在程序中调用时Delay_ms(18)和Delay_us(50)表示需要延时18毫秒和50微秒。
STM32F103C8T6的PB15做DHT11的数据传输口怎么配置程序
首先,需要在STM32F103C8T6的GPIO模块中对PB15进行配置。以下是一个简单的程序示例,以将PB15配置为输入模式:
```c
#include "stm32f10x.h"
int main(void)
{
// 使能PORTB时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// 配置PB15为输入模式
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_15;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU; // 选择内部上拉输入模式
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
while(1)
{
// 在此处添加程序逻辑
}
}
```
接下来,我们需要编写程序来读取DHT11传感器的数据。以下是一个简单的程序示例,用于读取DHT11传感器的湿度和温度数据:
```c
#include "stm32f10x.h"
#include "delay.h" // 这里使用了延时库,需要另外下载并添加到工程中
int main(void)
{
// 使能PORTB时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// 配置PB15为输入模式
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_15;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU; // 选择内部上拉输入模式
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
while(1)
{
// 发送起始信号
GPIO_ResetBits(GPIOB, GPIO_Pin_15);
delay_ms(18);
GPIO_SetBits(GPIOB, GPIO_Pin_15);
delay_us(40);
// 切换到输入模式
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPD; // 选择内部下拉输入模式
GPIO_Init(GPIOB, &GPIO_InitStructure);
// 等待DHT11响应
while(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_15) == SET);
// 等待DHT11发送数据
while(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_15) == RESET);
// 读取数据
uint8_t data[5] = {0};
for(int i=0; i<40; i++)
{
// 等待一个高电平
while(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_15) == RESET);
// 等待50us,然后判断是0还是1
delay_us(50);
if(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_15) == SET)
{
data[i/8] |= (1 << (7 - (i % 8)));
}
// 等待低电平结束
while(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_15) == SET);
}
// 切换到输出模式
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(GPIOB, &GPIO_InitStructure);
// 计算湿度和温度
uint8_t sum = data[0] + data[1] + data[2] + data[3];
if(sum == data[4])
{
uint16_t humidity = ((uint16_t)data[0] << 8) | data[1];
uint16_t temperature = ((uint16_t)data[2] << 8) | data[3];
// 在此处处理湿度和温度数据
}
// 延时一段时间后再次读取
delay_ms(2000);
}
}
```
需要注意的是,以上程序示例仅供参考,实际使用时需要根据DHT11传感器的具体数据协议进行调整。同时,需要根据实际情况选择合适的延时时间和GPIO模式,以确保正确读取数据。
阅读全文
相关推荐
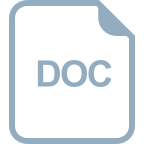
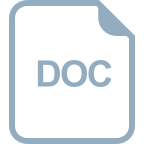
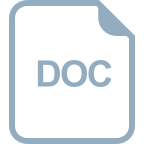
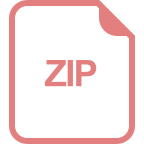
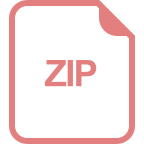

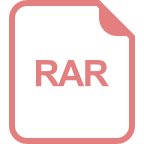


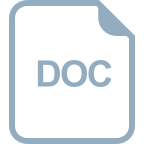
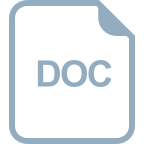
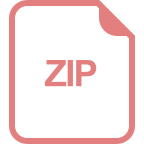
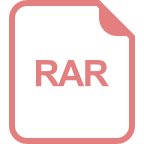
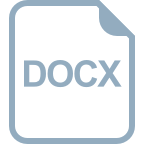
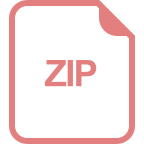