python调用dxgi截图
时间: 2023-09-05 09:09:59 浏览: 90
要在Python中调用DXGI截图,需要使用PyWin32库和Python for .NET库。下面是一个简单的示例代码,可以截取桌面的屏幕截图:
```python
import clr
import win32gui
import win32ui
import win32con
import win32api
import numpy as np
# Load required .NET assemblies
clr.AddReference('System.Drawing')
clr.AddReference('System.Windows.Forms')
from System.Drawing import Bitmap, Graphics
from System.Windows.Forms import Screen
# Get the primary screen
screen = Screen.PrimaryScreen
# Get the screen dimensions
left, top, right, bottom = screen.Bounds.Left, screen.Bounds.Top, screen.Bounds.Right, screen.Bounds.Bottom
# Get the device context handle for the entire screen
hdc_screen = win32gui.GetDC(0)
# Create a memory device context for the screen
hdc_mem = win32gui.CreateCompatibleDC(hdc_screen)
# Create a bitmap to hold the screenshot
bmp_screen = win32ui.CreateBitmap()
bmp_screen.CreateCompatibleBitmap(hdc_screen, right - left, bottom - top)
# Select the bitmap into the memory device context
win32gui.SelectObject(hdc_mem, bmp_screen.GetHandle())
# Copy the screen into the memory device context
win32gui.BitBlt(hdc_mem, 0, 0, right - left, bottom - top, hdc_screen, left, top, win32con.SRCCOPY)
# Convert the bitmap to a numpy array
bmp_array = np.frombuffer(bmp_screen.GetBitmapBits(True), dtype=np.uint8)
bmp_array = bmp_array.reshape((bottom - top, right - left, 4))
# Release the device contexts
win32gui.DeleteObject(bmp_screen.GetHandle())
win32gui.DeleteDC(hdc_mem)
win32gui.ReleaseDC(0, hdc_screen)
# Display the screenshot
import matplotlib.pyplot as plt
plt.imshow(bmp_array)
plt.show()
```
这个示例代码使用了Windows API函数BitBlt将屏幕截图复制到一个内存设备上下文中,然后使用PyWin32库和Python for .NET库将内存设备上下文中的位图转换为一个NumPy数组,最后使用Matplotlib库显示截图。
相关推荐
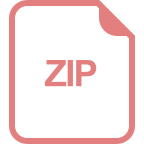














