利用python,写一个贪吃蛇的程序
时间: 2024-03-17 10:47:18 浏览: 56
以下是一个简单的贪吃蛇程序,基于Python的pygame库实现。程序中使用了一些常用的pygame函数,如`pygame.init()`初始化、`pygame.display.set_mode()`创建窗口、`pygame.draw.rect()`绘制矩形等。
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置窗口尺寸
WINDOW_SIZE = (400, 400)
screen = pygame.display.set_mode(WINDOW_SIZE)
# 设置游戏参数
BLOCK_SIZE = 10
SCORE_FONT = pygame.font.SysFont("arial", 20)
SNAKE_COLOR = (0, 255, 0)
FOOD_COLOR = (255, 0, 0)
# 定义Snake类
class Snake:
def __init__(self):
self.body = [(WINDOW_SIZE[0] // 2, WINDOW_SIZE[1] // 2)]
self.direction = (0, -1)
self.score = 0
# 更新蛇的状态
def update(self, food_pos):
# 更新蛇头位置
head = (self.body[0][0] + self.direction[0] * BLOCK_SIZE, self.body[0][1] + self.direction[1] * BLOCK_SIZE)
self.body.insert(0, head)
# 如果吃到了食物
if head == food_pos:
self.score += 1
else:
self.body.pop()
# 如果撞到了墙壁
if head[0] < 0 or head[0] >= WINDOW_SIZE[0] or head[1] < 0 or head[1] >= WINDOW_SIZE[1]:
return False
# 如果撞到了自己的身体
if head in self.body[1:]:
return False
return True
# 绘制蛇的身体
def draw(self, screen):
for pos in self.body:
pygame.draw.rect(screen, SNAKE_COLOR, (pos[0], pos[1], BLOCK_SIZE, BLOCK_SIZE))
# 改变蛇的方向
def change_direction(self, direction):
if direction == "up":
self.direction = (0, -1)
elif direction == "down":
self.direction = (0, 1)
elif direction == "left":
self.direction = (-1, 0)
elif direction == "right":
self.direction = (1, 0)
# 定义Food类
class Food:
def __init__(self):
self.pos = (random.randint(0, WINDOW_SIZE[0] // BLOCK_SIZE - 1) * BLOCK_SIZE,
random.randint(0, WINDOW_SIZE[1] // BLOCK_SIZE - 1) * BLOCK_SIZE)
# 绘制食物
def draw(self, screen):
pygame.draw.rect(screen, FOOD_COLOR, (self.pos[0], self.pos[1], BLOCK_SIZE, BLOCK_SIZE))
# 重新生成食物
def respawn(self):
self.pos = (random.randint(0, WINDOW_SIZE[0] // BLOCK_SIZE - 1) * BLOCK_SIZE,
random.randint(0, WINDOW_SIZE[1] // BLOCK_SIZE - 1) * BLOCK_SIZE)
# 初始化游戏
snake = Snake()
food = Food()
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
snake.change_direction("up")
elif event.key == pygame.K_DOWN:
snake.change_direction("down")
elif event.key == pygame.K_LEFT:
snake.change_direction("left")
elif event.key == pygame.K_RIGHT:
snake.change_direction("right")
# 更新蛇的状态
if not snake.update(food.pos):
pygame.quit()
print("Game Over!")
quit()
# 判断是否吃到了食物
if snake.body[0] == food.pos:
food.respawn()
# 绘制游戏界面
screen.fill((255, 255, 255))
food.draw(screen)
snake.draw(screen)
# 显示分数
score_text = SCORE_FONT.render("Score:{}".format(snake.score), True, (0, 0, 0))
screen.blit(score_text, (10, 10))
# 更新屏幕
pygame.display.update()
```
运行程序后,可以使用方向键来控制蛇的移动,蛇会不断地移动并尝试吃到食物。当蛇撞到墙壁或自己的身体时,游戏结束。程序还显示了当前的得分。
阅读全文
相关推荐
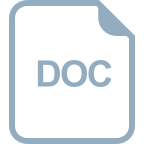
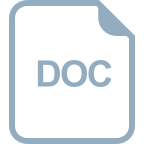
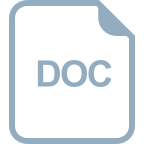

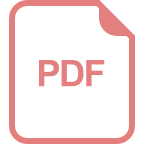
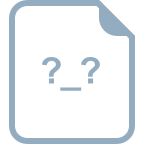
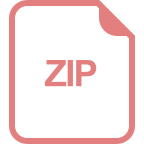
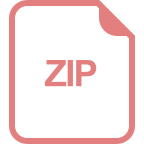
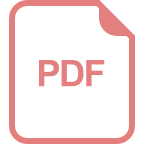



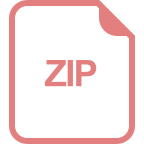
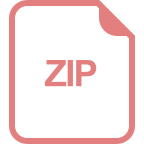
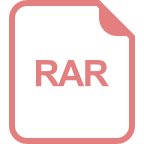
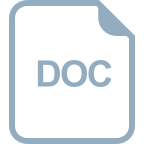
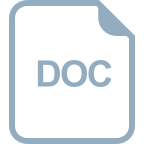
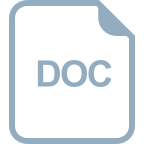