gps坐标解算,位置变换的一些操作算法代码用C++语言实现
时间: 2024-02-17 17:03:56 浏览: 108
这是一个比较复杂的问题,需要涉及到不同的算法和数学知识。以下是一个简单的 C++ 实现,可以作为参考:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const double pi = 3.14159265358979323846; // 圆周率
// 定义一个表示经纬度的结构体
struct Coordinate {
double longitude; // 经度
double latitude; // 纬度
};
// 计算两个经纬度之间的距离
double distance(Coordinate a, Coordinate b) {
double radLat1 = a.latitude * pi / 180.0;
double radLat2 = b.latitude * pi / 180.0;
double aLat = radLat1 - radLat2;
double aLon = (a.longitude - b.longitude) * pi / 180.0;
double s = 2 * asin(sqrt(pow(sin(aLat / 2), 2) + cos(radLat1) * cos(radLat2) * pow(sin(aLon / 2), 2)));
s = s * 6378.137;
s = round(s * 10000) / 10000; // 保留四位小数
return s;
}
// 计算两个经纬度之间的方位角
double bearing(Coordinate a, Coordinate b) {
double radLat1 = a.latitude * pi / 180.0;
double radLat2 = b.latitude * pi / 180.0;
double aLon = (b.longitude - a.longitude) * pi / 180.0;
double y = sin(aLon) * cos(radLat2);
double x = cos(radLat1) * sin(radLat2) - sin(radLat1) * cos(radLat2) * cos(aLon);
double brng = atan2(y, x);
brng = brng * 180 / pi;
brng = fmod(brng + 360, 360); // 将方位角转化为0-360度
return brng;
}
// 计算一个经纬度点的偏移量
Coordinate offset(Coordinate c, double brng, double dist) {
double radLat = c.latitude * pi / 180.0;
double radLon = c.longitude * pi / 180.0;
double d = dist / 6378.137;
double lat2 = asin(sin(radLat) * cos(d) + cos(radLat) * sin(d) * cos(brng));
double lon2 = radLon + atan2(sin(brng) * sin(d) * cos(radLat), cos(d) - sin(radLat) * sin(lat2));
lon2 = fmod((lon2 + 3 * pi), (2 * pi)) - pi; // 将经度转化为-180到180度
Coordinate result = { lon2 * 180 / pi, lat2 * 180 / pi };
return result;
}
int main() {
Coordinate a = { 116.404, 39.915 }; // 北京市中心
Coordinate b = { 121.473, 31.230 }; // 上海市中心
double dist = distance(a, b);
double brng = bearing(a, b);
cout << "距离: " << dist << " km" << endl;
cout << "方位角: " << brng << " 度" << endl;
Coordinate c = offset(a, brng, 100);
cout << "偏移点: (" << c.longitude << ", " << c.latitude << ")" << endl;
return 0;
}
```
这个程序实现了以下几个功能:
1. 计算两个经纬度之间的距离;
2. 计算两个经纬度之间的方位角;
3. 计算一个经纬度点的偏移量。
你可以根据自己的需求,对这个程序进行修改和扩展。
阅读全文
相关推荐
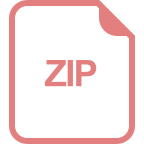
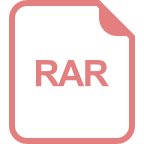
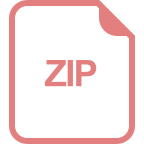
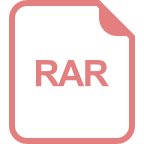
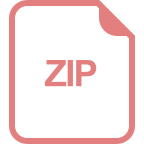
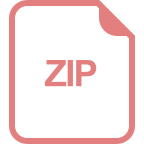
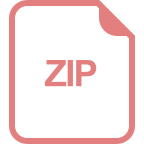
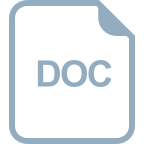
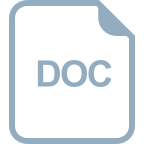

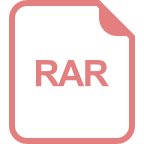
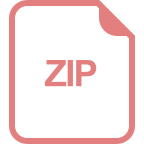
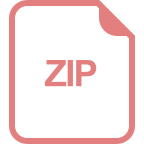
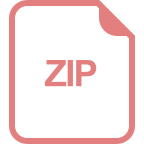
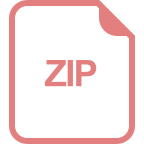
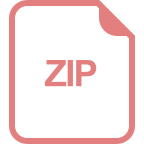