用java语言实现一个BER编解码器
时间: 2024-06-08 19:05:54 浏览: 119
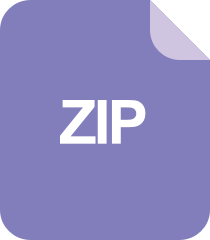
基于Java实现的ASN.1 BER 和 DER 编码解码库.zip
BER(Basic Encoding Rules,基本编码规则)是ASN.1(Abstract Syntax Notation One,抽象语法表示法一)的一种编码方式,其作用是将ASN.1类型数据编码为二进制格式,并且支持可扩展性。下面是一个简单的Java实现示例:
```java
import java.io.ByteArrayOutputStream;
import java.io.IOException;
public class BERCodec {
// 编码
public static byte[] encode(int tag, byte[] value) {
ByteArrayOutputStream out = new ByteArrayOutputStream();
try {
// 编码tag
int tagClass = tag & 0xC0;
int tagNumber = tag & 0x1F;
out.write(tagClass | tagNumber);
// 编码length
if (value.length < 128) {
out.write(value.length);
} else {
int lengthBytes = (Integer.SIZE - Integer.numberOfLeadingZeros(value.length)) / 7 + 1;
out.write(0x80 | lengthBytes);
for (int i = lengthBytes - 1; i >= 0; i--) {
out.write((value.length >> (i * 8)) & 0xFF);
}
}
// 编码value
out.write(value);
} catch (IOException e) {
e.printStackTrace();
}
return out.toByteArray();
}
// 解码
public static byte[] decode(byte[] data) {
ByteArrayOutputStream out = new ByteArrayOutputStream();
try {
// 解码tag
int tagClass = data[0] & 0xC0;
int tagNumber = data[0] & 0x1F;
int index = 1;
// 解码length
int length = data[index++] & 0xFF;
if ((length & 0x80) != 0) {
int lengthBytes = length & 0x7F;
length = 0;
for (int i = 0; i < lengthBytes; i++) {
length = (length << 8) | (data[index++] & 0xFF);
}
}
// 解码value
byte[] value = new byte[length];
System.arraycopy(data, index, value, 0, length);
out.write(value);
} catch (IOException e) {
e.printStackTrace();
}
return out.toByteArray();
}
}
```
这个实现只是一个简单的示例,仅支持tag值在0~31之间的BER编解码。对于更复杂的数据类型和更大的数据量,需要使用更完善的ASN.1库。
阅读全文
相关推荐
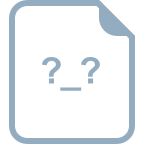


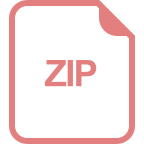
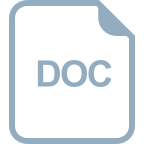
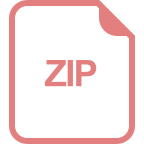
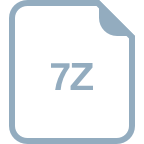
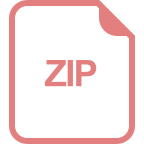
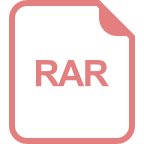
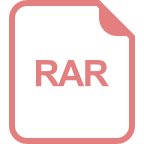
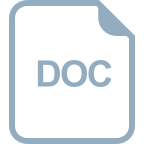
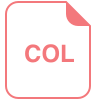
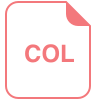
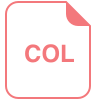
