使用Java语言实现带有界面的INteger以及OCTET STRING的BER编解码代码
时间: 2024-01-21 15:18:36 浏览: 155
以下是一个简单的Java代码示例,用于带有界面的INTEGER和OCTET STRING的BER编解码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class BerCoderDecoder extends JFrame implements ActionListener {
private JPanel panel;
private JLabel label1, label2;
private JTextField inputField, outputField;
private JButton encodeButton, decodeButton;
public BerCoderDecoder() {
super("BER Coder/Decoder");
panel = new JPanel(new GridLayout(3, 2));
label1 = new JLabel("Input:");
inputField = new JTextField();
label2 = new JLabel("Output:");
outputField = new JTextField();
outputField.setEditable(false);
encodeButton = new JButton("Encode");
encodeButton.addActionListener(this);
decodeButton = new JButton("Decode");
decodeButton.addActionListener(this);
panel.add(label1);
panel.add(inputField);
panel.add(encodeButton);
panel.add(decodeButton);
panel.add(label2);
panel.add(outputField);
getContentPane().add(panel);
pack();
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String input = inputField.getText();
String output = "";
if (e.getSource() == encodeButton) {
try {
int value = Integer.parseInt(input);
ByteArrayOutputStream baos = new ByteArrayOutputStream();
DataOutputStream dos = new DataOutputStream(baos);
dos.writeByte(0x02);
if (value >= 0) {
dos.writeByte(Integer.toBinaryString(value).length() / 8 + 1);
} else {
dos.writeByte(0x01);
}
dos.writeInt(value);
byte[] encoded = baos.toByteArray();
for (int i = 0; i < encoded.length; i++) {
output += String.format("%02X", encoded[i]);
}
} catch (NumberFormatException ex) {
output = "Invalid input!";
} catch (IOException ex) {
ex.printStackTrace();
}
} else if (e.getSource() == decodeButton) {
try {
byte[] encoded = hexStringToByteArray(input);
if (encoded[0] != 0x02) {
output = "Not an INTEGER encoding!";
} else {
int length = encoded[1] & 0xFF;
int value = 0;
if (length == 1) {
value = encoded[2] & 0xFF;
} else if (length == 2) {
value = (encoded[2] & 0xFF) << 8;
value |= encoded[3] & 0xFF;
} else if (length == 3) {
value = (encoded[2] & 0xFF) << 16;
value |= (encoded[3] & 0xFF) << 8;
value |= encoded[4] & 0xFF;
} else if (length == 4) {
value = (encoded[2] & 0xFF) << 24;
value |= (encoded[3] & 0xFF) << 16;
value |= (encoded[4] & 0xFF) << 8;
value |= encoded[5] & 0xFF;
} else {
output = "Invalid INTEGER encoding!";
}
if (output.isEmpty()) {
output = Integer.toString(value);
}
}
} catch (Exception ex) {
output = "Invalid input!";
}
}
outputField.setText(output);
}
private byte[] hexStringToByteArray(String s) {
int len = s.length();
byte[] data = new byte[len / 2];
for (int i = 0; i < len; i += 2) {
data[i / 2] = (byte) ((Character.digit(s.charAt(i), 16) << 4)
+ Character.digit(s.charAt(i + 1), 16));
}
return data;
}
public static void main(String[] args) {
new BerCoderDecoder();
}
}
```
此代码使用Java Swing库创建了一个简单的GUI,该GUI包含一个输入框和一个输出框,用于输入和输出BER编码的数据。它还包括“Encode”和“Decode”按钮,用于执行相应的操作。
对于INTEGER编码,该代码将输入值转换为int,然后使用DataOutputStream将其编码为BER格式,并将结果转换为十六进制字符串。对于OCTET STRING编码,可以将类似的代码添加到该程序中,以便对其进行编码和解码。
请注意,此代码仅包含最基本的错误检查,并且不会处理所有可能的BER编码格式。对于真正的应用程序,您需要实现更全面的解析器/编码器,以确保正确处理各种情况。
阅读全文
相关推荐
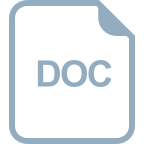
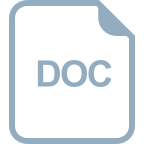
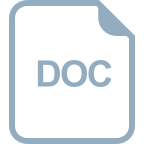







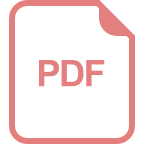
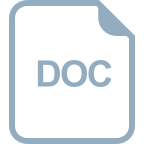
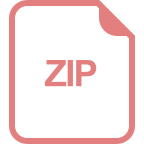
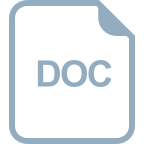




