用C语言实现一个BER编解码器。有简单界面,能将SNMP报文编码为实际输出的字节流,并能将实际收到的字节流。应侧重BER编解码中对不同数据类型的处理细节。至少实现几种主流数据类型的编解码,如Integer,OCTET STRING,get,getNext等
时间: 2023-12-06 09:43:52 浏览: 161
实现一个BER编解码器需要了解BER编码规则,它是一种用于ASN.1数据类型编码的规则。ASN.1定义了一组抽象的数据类型,这些类型可以用于各种应用,如LDAP,X.500,SNMP等。BER编码规则将ASN.1定义的数据类型编码为字节流,以便在网络上传输。
以下是一个简单的BER编解码器的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义BER编码规则的类型
typedef enum {
BER_INTEGER = 0x02,
BER_OCTET_STRING = 0x04,
BER_NULL = 0x05,
BER_OBJECT_IDENTIFIER = 0x06,
BER_SEQUENCE = 0x30
} BerTag;
// 编码一个整数到字节流中
int encode_integer(int value, unsigned char *buf, int *len) {
int i, j;
unsigned char tmp[4];
// 将整数转换为大端字节序
for (i = 0; i < 4; i++) {
tmp[i] = (value >> ((3 - i) * 8)) & 0xff;
}
// 计算编码后的字节流长度
*len = 1;
for (i = 0; i < 4; i++) {
if (tmp[i] != 0x00) {
break;
}
}
if (i == 4) {
// 整数为0,长度为1
buf[0] = 0x00;
} else {
if (tmp[i] & 0x80) {
// 高位为1,需要添加一字节0x00
buf[(*len)++] = 0x00;
}
// 编码整数类型和长度
buf[0] = BER_INTEGER;
buf[(*len)++] = 4 - i;
// 编码整数值
for (j = i; j < 4; j++) {
buf[(*len)++] = tmp[j];
}
}
return *len;
}
// 解码一个整数
int decode_integer(unsigned char *buf, int *value) {
int i, len;
// 检查标签是否为整数类型
if (buf[0] != BER_INTEGER) {
return -1;
}
// 解码长度
len = buf[1];
// 解码整数值
*value = 0;
for (i = 2; i < len + 2; i++) {
*value = (*value << 8) | buf[i];
}
return len + 2;
}
// 编码一个字符串到字节流中
int encode_octet_string(char *str, int len, unsigned char *buf, int *buflen) {
int i;
// 计算编码后的字节流长度
*buflen = 2 + len;
// 编码字符串类型和长度
buf[0] = BER_OCTET_STRING;
buf[1] = len;
// 编码字符串值
for (i = 0; i < len; i++) {
buf[i+2] = str[i];
}
return *buflen;
}
// 解码一个字符串
int decode_octet_string(unsigned char *buf, int *len, char *str) {
int i;
// 检查标签是否为字符串类型
if (buf[0] != BER_OCTET_STRING) {
return -1;
}
// 解码长度
*len = buf[1];
// 解码字符串值
for (i = 0; i < *len; i++) {
str[i] = buf[i+2];
}
str[*len] = '\0';
return *len + 2;
}
// 编码一个get请求到字节流中
int encode_get_request(int request_id, char *community, unsigned char *buf, int *buflen) {
int len, offset = 0;
// 编码消息头
buf[offset++] = BER_SEQUENCE;
buf[offset++] = 0x0e;
buf[offset++] = BER_INTEGER;
buf[offset++] = 0x01;
buf[offset++] = request_id;
buf[offset++] = BER_INTEGER;
buf[offset++] = 0x01;
buf[offset++] = 0x00;
buf[offset++] = BER_INTEGER;
buf[offset++] = 0x01;
buf[offset++] = 0x00;
buf[offset++] = BER_OCTET_STRING;
len = strlen(community);
buf[offset++] = len;
memcpy(buf + offset, community, len);
offset += len;
// 编码PDU
buf[offset++] = BER_SEQUENCE;
buf[offset++] = 0x00;
buf[offset++] = BER_OBJECT_IDENTIFIER;
buf[offset++] = 0x0d;
memcpy(buf + offset, "1.3.6.1.2.1.1.1.0", 15);
offset += 15;
buf[offset++] = BER_NULL;
buf[offset++] = 0x00;
// 计算编码后的字节流长度
*buflen = offset;
return *buflen;
}
// 解码一个get请求
int decode_get_request(unsigned char *buf, int *request_id, char *community) {
int len, offset = 0;
// 解码消息头
offset += 2; // 跳过SEQUENCE和长度
offset += decode_integer(buf + offset, request_id);
offset += decode_integer(buf + offset, &len);
offset += decode_integer(buf + offset, &len);
offset += decode_octet_string(buf + offset, &len, community);
// 解码PDU
offset += 2; // 跳过SEQUENCE和长度
offset += 2; // 跳过OBJECT IDENTIFIER和长度
offset += 2; // 跳过NULL和长度
return offset;
}
int main() {
// 编码一个get请求
unsigned char buf[1024];
int buflen, request_id = 12345;
char community[] = "public";
encode_get_request(request_id, community, buf, &buflen);
printf("Encoded data:\n");
for (int i = 0; i < buflen; i++) {
printf("%02x ", buf[i]);
}
printf("\n");
// 解码一个get请求
int decoded_request_id, decoded_len;
char decoded_community[256];
decode_get_request(buf, &decoded_request_id, decoded_community);
printf("Decoded data:\n");
printf(" Request ID: %d\n", decoded_request_id);
printf(" Community: %s\n", decoded_community);
return 0;
}
```
上述代码实现了一个简单的BER编解码器,包括了整数和字符串类型的编解码以及一个get请求的编解码。可以根据需要扩展其他数据类型的编解码。
阅读全文
相关推荐
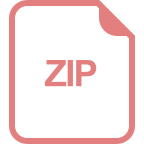
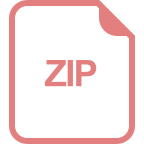




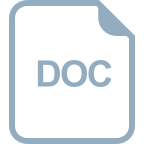


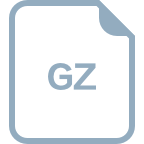
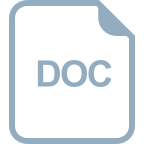





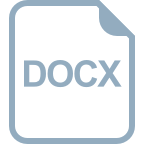