用windows c++ 写一个代码, 可以遍历一个目录中的文本文件, 如果文件的编码是utf8, 那么不进行变化, 如果文本编码不是utf8, 那么将文本转换为utf8的编码格式
时间: 2024-11-22 21:39:36 浏览: 16
在Windows上使用C++编写代码来遍历目录、检测文件编码并转换成UTF-8格式,这需要涉及一些操作系统操作、文件I/O以及字符编码处理库,例如`<filesystem>`库(C++17及以上),以及可能的第三方库如`iconv`或者`ICU`来进行编码转换。下面是一个简单的示例代码框架:
```cpp
#include <iostream>
#include <filesystem>
#include <locale>
#include <codecvt>
// 假设我们使用宽字符(wide character)来处理非ASCII字符
std::wstring_convert<std::codecvt_utf8<wchar_t>> utf8_to_wchar;
void convert_to_utf8(const std::string& filename) {
if (std::filesystem::is_regular_file(filename)) {
// 打开文件
std::ifstream file(filename, std::ios::binary);
// 检查文件是否能以UTF-8读取(假设文件头标识了其编码)
char header[4] = {0};
file.read(header, sizeof(header));
if (std::memcmp(header, "\xEF\xBB\xBF", 3) == 0) { // UTF-8 BOM
// 文件已经是UTF-8,直接读取内容
std::string content((std::istreambuf_iterator<char>(file)), {});
} else {
// 非UTF-8,尝试转换
try {
std::wstring wstr_content;
file.rdbuf()->pubsetbuf(nullptr, 0); // 预读取所有数据到内存
file >> wstr_content; // 转换读入的内容
// 使用转换器将宽字符转为UTF-8字符串
std::string converted_content(utf8_to_wchar.from_bytes(wstr_content));
// 写回转换后的UTF-8文件,这里省略了实际操作
std::ofstream utf8_file(filename, std::ios::binary);
utf8_file << converted_content;
}
catch (...) {
std::cerr << "Failed to convert file " << filename << std::endl;
}
}
file.close();
}
}
int main() {
for (const auto& entry : std::filesystem::directory_iterator("path/to/directory")) {
if (entry.is_regular_file()) {
convert_to_utf8(entry.path().string());
}
}
return 0;
}
```
注意,这个示例假设文件头存在BOM(Byte Order Mark)来标记UTF-8编码。实际情况中,你可能需要采用其他方法(例如检查文件头部字节序列或者依赖于特定的库)来确定文件的实际编码。另外,这个例子只演示了基本的转换流程,实际应用中你可能还需要考虑错误处理和性能优化。
阅读全文
相关推荐
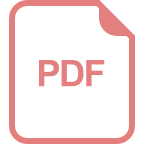
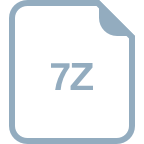
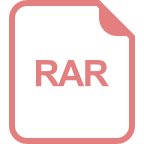


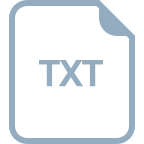
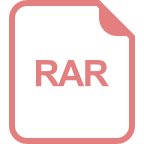
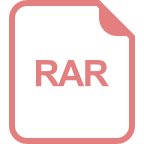
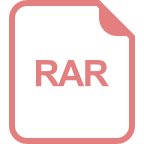
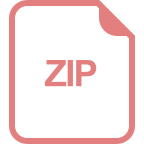
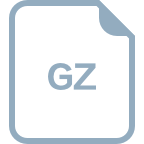
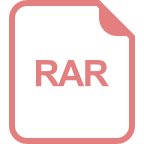
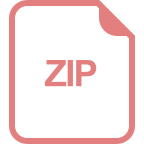
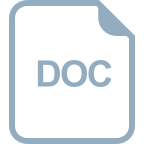
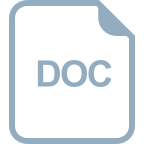
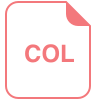


