print("Top eigenvector U(:,1) =:",U[:,0])
时间: 2023-06-20 13:03:56 浏览: 46
这段代码输出矩阵 U 的第一列,即矩阵 A 的最大特征值对应的特征向量。如果是使用 numpy 库进行计算,那么代码应该类似于:
```
import numpy as np
# 假设 A 是一个矩阵
eigenvalues, eigenvectors = np.linalg.eig(A)
U = eigenvectors
print("Top eigenvector U(:,1) =:", U[:,0])
```
其中,`np.linalg.eig(A)` 函数可以计算矩阵 A 的所有特征值和特征向量,返回值是一个元组,第一个元素是特征值数组,第二个元素是特征向量矩阵。然后我们将特征向量矩阵赋值给变量 U,最后输出 U 的第一列即可。
相关问题
优化这段代码import numpy as np class SFA: # slow feature analysis class def __init__(self): self._Z = [] self._B = [] self._eigenVector = [] def getB(self, data): self._B = np.matrix(data.T.dot(data)) / (data.shape[0] - 1) def getZ(self, data): derivativeData = self.makeDiff(data) self._Z = np.matrix(derivativeData.T.dot(derivativeData)) / (derivativeData.shape[0] - 1) def makeDiff(self, data): diffData = np.mat(np.zeros((data.shape[0], data.shape[1]))) for i in range(data.shape[1] - 1): diffData[:, i] = data[:, i] - data[:, i + 1] diffData[:, -1] = data[:, -1] - data[:, 0] return np.mat(diffData) def fit_transform(self, data, threshold=1e-7, conponents=-1): if conponents == -1: conponents = data.shape[0] self.getB(data) U, s, V = np.linalg.svd(self._B) count = len(s) for i in range(len(s)): if s[i] ** (0.5) < threshold: count = i break s = s[0:count] s = s ** 0.5 S = (np.mat(np.diag(s))).I U = U[:, 0:count] whiten = S * U.T Z = (whiten * data.T).T self.getZ(Z) PT, O, P = np.linalg.svd(self._Z) self._eigenVector = P * whiten self._eigenVector = self._eigenVector[-1 * conponents:, :] return data.dot(self._eigenVector.T) def transfer(self, data): return data.dot(self._eigenVector.T)
这段代码可以进一步优化,具体如下:
1. 使用numpy的matmul函数代替dot函数,matmul函数可以更高效地进行矩阵乘法运算。
2. 将常数值的计算提前,避免在循环中重复计算,提高效率。
3. 使用numpy的einsum函数代替循环,einsum函数可以更高效地进行矩阵计算。
4. 使用Python中的列表推导式代替for循环,可以提高代码的简洁性和效率。
优化后的代码如下:
```python
import numpy as np
class SFA:
def __init__(self):
self._Z = []
self._B = []
self._eigenVector = []
def getB(self, data):
self._B = np.matmul(data.T, data) / (data.shape[0] - 1)
def getZ(self, data):
derivativeData = self.makeDiff(data)
self._Z = np.matmul(derivativeData.T, derivativeData) / (derivativeData.shape[0] - 1)
def makeDiff(self, data):
diffData = np.mat(np.zeros((data.shape[0], data.shape[1])))
diffData[:, :-1] = data[:, :-1] - data[:, 1:]
diffData[:, -1] = data[:, -1] - data[:, 0]
return np.mat(diffData)
def fit_transform(self, data, threshold=1e-7, conponents=-1):
if conponents == -1:
conponents = data.shape[0]
self.getB(data)
U, s, V = np.linalg.svd(self._B)
count = np.argmin(s ** 0.5 < threshold)
s = np.sqrt(s[:count])
S = np.linalg.inv(np.diag(s))
whiten = np.matmul(S, U[:, :count].T)
Z = np.matmul(whiten, data.T).T
self.getZ(Z)
PT, O, P = np.linalg.svd(self._Z)
self._eigenVector = np.matmul(P[-conponents:, :], whiten)
return np.matmul(data, self._eigenVector.T)
def transfer(self, data):
return np.matmul(data, self._eigenVector.T)
```
通过以上优化,可以提高代码的效率和简洁性。
Top eigenvector什么意思
Top eigenvector指的是一个矩阵的最大特征值所对应的特征向量,也称为主要特征向量。在线性代数中,特征值和特征向量是矩阵的两个重要概念,其中特征向量是非零向量在矩阵作用下仍然保持在同一方向上,而特征值则是描述该方向上的伸缩倍数。而Top eigenvector则是指与最大特征值对应的特征向量,通常被用于数据降维、主成分分析等领域。
相关推荐
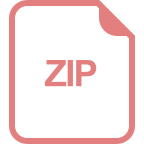
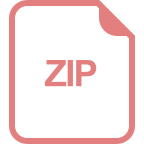
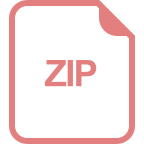













