用c语言解决三个消费者三个生产者十个缓冲区的问题,用一个信号量A来记录为空的缓冲区个数,另一个信号量B记录非空的缓冲区个数,然后生产者等待信号量A,消费者等待信号量B
时间: 2024-05-14 07:19:28 浏览: 119
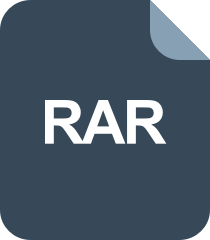
信号量来解决操作系统的消费者与生产者问题.rar_信号量_生产者 消费者_进程
好的,下面是一个简单的三个消费者三个生产者十个缓冲区的问题的解决方案,使用C语言和信号量实现。
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 10
#define NUM_PRODUCERS 3
#define NUM_CONSUMERS 3
int buffer[BUFFER_SIZE];
sem_t empty; // 记录空缓冲区个数
sem_t full; // 记录非空缓冲区个数
pthread_mutex_t mutex; // 互斥锁,保证对缓冲区的访问不会发生冲突
int in = 0, out = 0; // 缓冲区的写入和读取位置
void *producer(void *arg) {
int item, i;
for (i = 0; i < BUFFER_SIZE; i++) {
item = rand() % BUFFER_SIZE; // 生产一个随机数
sem_wait(&empty); // 等待空缓冲区
pthread_mutex_lock(&mutex); // 获取互斥锁
buffer[in] = item; // 将item写入缓冲区
in = (in + 1) % BUFFER_SIZE; // 更新写入位置
printf("producer %d produced item %d\n", *(int *)arg, item);
pthread_mutex_unlock(&mutex); // 释放互斥锁
sem_post(&full); // 增加非空缓冲区个数
}
return NULL;
}
void *consumer(void *arg) {
int item, i;
for (i = 0; i < BUFFER_SIZE; i++) {
sem_wait(&full); // 等待非空缓冲区
pthread_mutex_lock(&mutex); // 获取互斥锁
item = buffer[out]; // 从缓冲区读取item
out = (out + 1) % BUFFER_SIZE; // 更新读取位置
printf("consumer %d consumed item %d\n", *(int *)arg, item);
pthread_mutex_unlock(&mutex); // 释放互斥锁
sem_post(&empty); // 增加空缓冲区个数
}
return NULL;
}
int main() {
pthread_t producer_thread[NUM_PRODUCERS];
pthread_t consumer_thread[NUM_CONSUMERS];
sem_init(&empty, 0, BUFFER_SIZE); // 初始化空缓冲区个数为BUFFER_SIZE
sem_init(&full, 0, 0); // 初始化非空缓冲区个数为0
pthread_mutex_init(&mutex, NULL); // 初始化互斥锁
int i, producer_id[NUM_PRODUCERS], consumer_id[NUM_CONSUMERS];
for (i = 0; i < NUM_PRODUCERS; i++) {
producer_id[i] = i + 1;
pthread_create(&producer_thread[i], NULL, producer, &producer_id[i]);
}
for (i = 0; i < NUM_CONSUMERS; i++) {
consumer_id[i] = i + 1;
pthread_create(&consumer_thread[i], NULL, consumer, &consumer_id[i]);
}
for (i = 0; i < NUM_PRODUCERS; i++) {
pthread_join(producer_thread[i], NULL);
}
for (i = 0; i < NUM_CONSUMERS; i++) {
pthread_join(consumer_thread[i], NULL);
}
sem_destroy(&empty);
sem_destroy(&full);
pthread_mutex_destroy(&mutex);
return 0;
}
```
在这个程序中,我们创建了三个生产者线程和三个消费者线程。在 `producer` 函数中,每个生产者会生成一个随机数,并将其写入到缓冲区中。在 `consumer` 函数中,每个消费者会从缓冲区中读取一个 item 并打印出来。
我们使用了两个信号量 `empty` 和 `full` 来记录空缓冲区和非空缓冲区的个数。当缓冲区为空时,生产者需要等待 `empty` 信号量,表示有空缓冲区可以写入。当缓冲区非空时,消费者需要等待 `full` 信号量,表示有非空缓冲区可以读取。每次写入或读取一个 item 时,都需要获取互斥锁 `mutex`,以保证对缓冲区的访问不会发生冲突。
在主函数中,我们等待所有的生产者和消费者线程都运行结束后,释放信号量和互斥锁。
阅读全文
相关推荐
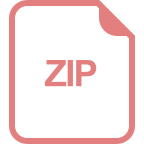















