dijkstra算法c语音代码
时间: 2024-11-05 09:11:23 浏览: 14
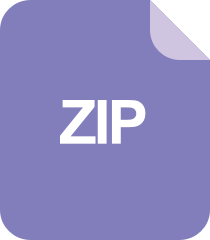
Dijkstra算法求最短路径代码.zip
Dijkstra算法的C语言实现可以帮助我们找到图中从给定起点到其他顶点的最短路径。以下是简单的Dijkstra算法在C语言中的基本步骤[^1]:
1. 初始化:创建一个优先队列(通常使用最小堆)并设置起点的距离为0,其余节点的距离设为无穷大。
```c
struct Node {
int distance;
int vertex;
};
// 初始化数组
priority_queue<Node*, vector<Node*>, greater<Node*>> pq;
pq.push({0, start});
```
2. 主循环:每次取出距离当前已知最短路径最近的节点,更新其相邻节点的距离。
```c
while (!pq.empty()) {
Node* current = pq.top();
pq.pop();
// 更新邻接节点的距离
if (current->distance < shortest_distances[current->vertex]) {
shortest_distances[current->vertex] = current->distance;
update_neighbors(current->vertex);
}
}
```
3. 更新邻居:对于每个未访问过的邻居,如果通过当前节点到达它们的距离更小,就更新这个邻居的距离并将它放入优先队列。
```c
void update_neighbors(int u) {
for (int v : adj[u]) {
int alt = shortest_distances[u] + weight(u, v);
if (alt < shortest_distances[v]) {
pq.push({alt, v});
}
}
}
```
其中`adj[u]`表示节点u的邻接节点集合,`weight(u, v)`表示从u到v的边的权重。
请注意,这个代码片段简化了一些细节,如数据结构的选择、错误处理以及遍历图的方式。实际应用中可能需要根据具体需求调整。
阅读全文
相关推荐
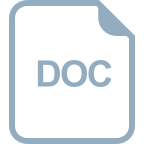
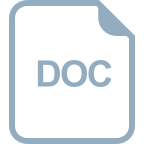

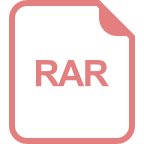

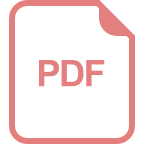

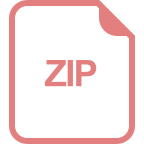
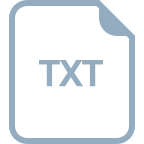
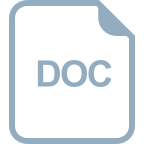



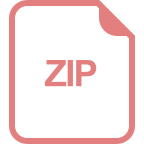
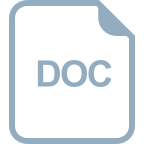


