用C++写代码:词法分析:【问题描述】拆分并识别输入的源代码文件中的单词。 【输入形式】存储于source.txt文件中的源代码。 【输出形式】存储单词的token.txt文件,以及存储标识符和常数的symbol.txt文件。
时间: 2024-10-17 15:07:50 浏览: 49
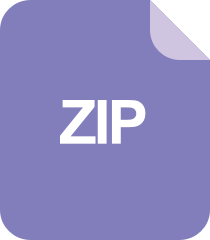
代码_词法分析_,词法分析程序,C,C++源码.zip
在C++中,词法分析通常是自然语言处理的第一步,它会将输入的源代码分割成一个个有意义的元素,如关键字、标识符、运算符和常量等。这通常通过创建一个简单的解析器或者使用现成的库来完成。这里是一个简化的示例,使用C++标准库`fstream`读取文件,并利用`istringstream`逐行分割:
```cpp
#include <iostream>
#include <fstream>
#include <sstream>
#include <string>
#include <map>
// 定义一些基本的词法类别
enum LexemeType {
KEYWORD,
IDENTIFIER,
CONSTANT,
OPERATOR,
COMMENT
};
class Tokenizer {
public:
void tokenize(const std::string& filename) {
std::ifstream input(filename);
if (!input.is_open()) {
std::cerr << "Failed to open file: " << filename << std::endl;
return;
}
std::string line;
while (std::getline(input, line)) {
processLine(line);
}
input.close();
}
private:
void processLine(const std::string& line) {
std::istringstream iss(line);
std::string token;
while (iss >> token) {
handleToken(token);
}
}
void handleToken(const std::string& token) {
// 这里需要实现具体的词法分析规则,比如检查是否是关键字、标识符、数字等等
// 示例:
if (isKeyword(token)) {
output.write("KEYWORD", 6); // 写入文件,这里的"KEYWORD"只是一个占位符
} else if (isIdentifier(token)) {
output.write("IDENTIFIER", 10); // 同理
} else if (isConstant(token)) {
output.write("CONSTANT", 8);
} else if (isOperator(token)) {
output.write("OPERATOR", 7);
} else if (token.find("//") == 0) { // 注释
output.write("COMMENT", 7);
} else {
std::cerr << "Unknown token: " << token << std::endl;
}
}
// 这些函数需要实际的实现,这里仅作示意
bool isKeyword(const std::string& token) { /* ... */ }
bool isIdentifier(const std::string& token) { /* ... */ }
bool isConstant(const std::string& token) { /* ... */ }
bool isOperator(const std::string& token) { /* ... */ }
std::ofstream output{"token.txt"};
};
int main() {
Tokenizer tokenizer;
tokenizer.tokenize("source.txt");
return 0;
}
阅读全文
相关推荐
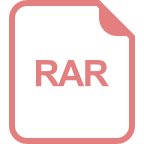
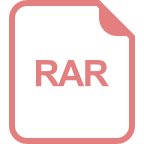
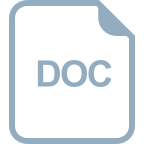
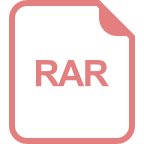
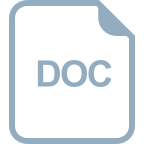
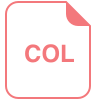
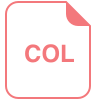


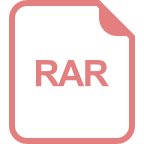
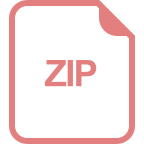
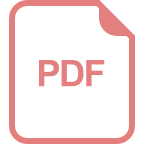
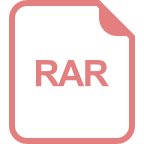
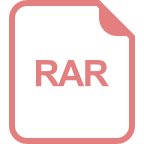
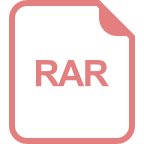
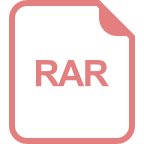
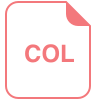
