stm32 串口接收
时间: 2023-10-19 10:09:26 浏览: 37
在 STM32 中,串口接收可以通过中断或者轮询的方式实现。其中,中断方式相对于轮询方式更加高效和可靠。下面是一个使用中断方式实现串口接收的示例代码:
```c
#include "stm32f4xx.h"
#define BUFFER_SIZE 100
uint8_t rx_buffer[BUFFER_SIZE];
uint8_t rx_index = 0;
void USART2_IRQHandler(void)
{
if (USART_GetITStatus(USART2, USART_IT_RXNE) != RESET)
{
rx_buffer[rx_index++] = USART_ReceiveData(USART2);
if (rx_index == BUFFER_SIZE)
{
rx_index = 0;
}
}
}
int main(void)
{
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
// Enable USART2 clock
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
// Configure USART2 pins
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Connect USART2 pins to AF7
GPIO_PinAFConfig(GPIOA, GPIO_PinSource2, GPIO_AF_USART2);
GPIO_PinAFConfig(GPIOA, GPIO_PinSource3, GPIO_AF_USART2);
// Configure USART2
USART_StructInit(&USART_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_Init(USART2, &USART_InitStructure);
// Enable USART2 RX interrupt
USART_ITConfig(USART2, USART_IT_RXNE, ENABLE);
// Configure NVIC
NVIC_InitStructure.NVIC_IRQChannel = USART2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0; NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
// Enable USART2
USART_Cmd(USART2, ENABLE);
while (1)
{
// Do something else
}
}
```
在上面的代码中,我们首先定义了一个大小为 BUFFER_SIZE 的接收缓冲区 rx_buffer 和一个指向缓冲区当前位置的索引 rx_index。然后,在 USART2_IRQHandler 中,我们检查是否接收到了数据,并将其存储到接收缓冲区中。最后,在 main 函数中,我们启用了 USART2 和中断,并进入了一个无限循环中。
相关推荐
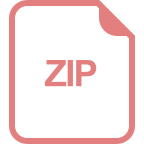
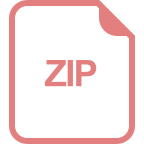














