python cv2 scale
时间: 2023-10-04 14:08:17 浏览: 61
在Python的OpenCV库中,可以使用cv2.resize()函数来进行图像的缩放。通过指定缩放后的图像大小,可以将原始图像缩放到所需的尺寸。下面是一个示例代码:
```python
import cv2
image = cv2.imread("D:/cv2Demo/lotus2.png") # 读取图像
width = int(image.shape[1] * 0.5) # 缩放后的宽度
height = int(image.shape[0] * 0.5) # 缩放后的高度
resized_image = cv2.resize(image, (width, height)) # 缩放图像
cv2.imwrite("D:/cv2Demo/lotus2Quarter.png", resized_image) # 保存缩放后的图像
```
这段代码将读取名为"lotus2.png"的图像,然后将其缩小为原始图像宽度和高度的一半,并将缩放后的图像保存为"lotus2Quarter.png"。
相关问题
python cv2库具体用法
Python的OpenCV库(cv2)是一个流行的计算机视觉库,它包含了许多用于图像处理和计算机视觉的函数。下面是一些cv2库的用法:
1. 读取图像
```
import cv2
img = cv2.imread('image_path')
```
2. 显示图像
```
import cv2
img = cv2.imread('image_path')
cv2.imshow('image',img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
3. 保存图像
```
import cv2
img = cv2.imread('image_path')
cv2.imwrite('output_image_path',img)
```
4. 图像缩放
```
import cv2
img = cv2.imread('image_path')
resized_img = cv2.resize(img,(new_width,new_height))
```
5. 图像旋转
```
import cv2
img = cv2.imread('image_path')
rows,cols = img.shape[:2]
M = cv2.getRotationMatrix2D((cols/2,rows/2),angle,scale)
rotated_img = cv2.warpAffine(img,M,(cols,rows))
```
这些仅仅是cv2库的一部分用法,还有许多其他功能可以探索。
python手写cv2.resize
cv2.resize()是OpenCV库中用于调整图像大小的函数。它可以通过手写代码来实现,以下是一个简单的示例:
```python
import numpy as np
def resize(image, new_size):
# 获取原始图像的尺寸
height, width = image.shape[:2]
new_height, new_width = new_size
# 计算宽高缩放比例
scale_x = new_width / width
scale_y = new_height / height
# 创建一个新的空白图像
resized_image = np.zeros((new_height, new_width, 3), dtype=np.uint8)
# 遍历新图像的每个像素
for y in range(new_height):
for x in range(new_width):
# 计算原始图像中对应的坐标
src_x = int(x / scale_x)
src_y = int(y / scale_y)
# 将原始图像的像素值赋给新图像
resized_image[y, x] = image[src_y, src_x]
return resized_image
```
这个函数接受两个参数:原始图像和目标尺寸。它首先计算宽高缩放比例,然后创建一个新的空白图像。接下来,它遍历新图像的每个像素,并根据缩放比例计算出对应的原始图像坐标,将原始图像的像素值赋给新图像。最后,返回调整大小后的图像。
请注意,这只是一个简单的手写示例,实际使用中可能需要考虑更多的细节和优化。另外,OpenCV库本身已经提供了cv2.resize()函数,可以直接使用该函数进行图像大小调整。
相关推荐
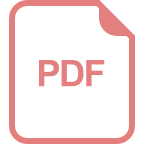
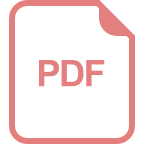
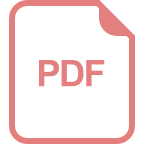












