三个模拟量通过PCF8591进行AD转换,并把三个数字量通过uart串口在电脑上显示,Verilog
时间: 2023-11-27 19:55:05 浏览: 84
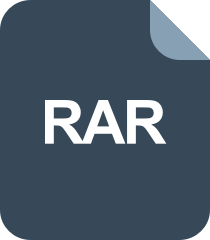
基于verilog实现的AD 转换程序

以下是一种可能的Verilog代码实现:
```
module PCF8591(
input wire clk,
input wire rst_n,
input wire start_conversion,
output reg [7:0] adc_result
);
reg [1:0] state;
reg [7:0] adc_data;
parameter IDLE = 2'b00;
parameter READ_ADC = 2'b01;
parameter SEND_UART = 2'b10;
// PCF8591 I2C bus control signals
reg sda;
reg scl;
reg ack;
reg [7:0] i2c_addr;
reg [7:0] i2c_data;
reg i2c_write;
// UART control signals
reg tx_busy;
reg [7:0] tx_data;
reg [7:0] tx_count;
// ADC control signals
reg adc_clk;
// FSM state transitions
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
state <= IDLE;
end else begin
case (state)
IDLE: begin
if (start_conversion) begin
state <= READ_ADC;
end else begin
state <= IDLE;
end
end
READ_ADC: begin
state <= SEND_UART;
end
SEND_UART: begin
if (tx_busy) begin
state <= SEND_UART;
end else begin
state <= IDLE;
end
end
default: begin
state <= IDLE;
end
endcase
end
end
// I2C bus state machine
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
sda <= 1'b1;
scl <= 1'b1;
ack <= 1'b1;
i2c_addr <= 8'h00;
i2c_data <= 8'h00;
i2c_write <= 1'b1;
end else begin
case (state)
IDLE: begin
// do nothing
end
READ_ADC: begin
if (adc_clk) begin
// start I2C write transaction to PCF8591
sda <= 1'b0;
i2c_addr <= 8'h90;
i2c_data <= 8'h00;
i2c_write <= 1'b1;
ack <= 1'b0;
end else begin
// finish I2C write transaction
sda <= 1'b1;
scl <= 1'b1;
i2c_write <= 1'b0;
ack <= 1'b1;
end
end
SEND_UART: begin
if (tx_busy) begin
// do nothing
end else begin
// start I2C read transaction from PCF8591
sda <= 1'b0;
i2c_addr <= 8'h91;
i2c_write <= 1'b0;
ack <= 1'b0;
end
end
default: begin
// do nothing
end
endcase
end
end
// I2C bus data transfer
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
// do nothing
end else begin
if (ack == 1'b0) begin
// send i2c_addr or i2c_data
if (i2c_write) begin
// send i2c_addr
sda <= i2c_addr[7];
i2c_addr <= i2c_addr << 1;
end else begin
// send i2c_data
sda <= i2c_data[7];
i2c_data <= i2c_data << 1;
end
end else begin
// receive i2c_data
sda <= 1'b1;
if (scl == 1'b0) begin
i2c_data <= {i2c_data[6:0], sda};
end
end
end
end
// ADC clock generation
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
adc_clk <= 1'b0;
end else begin
case (state)
IDLE: begin
adc_clk <= 1'b0;
end
READ_ADC: begin
if (adc_clk) begin
adc_data <= i2c_data;
end
adc_clk <= ~adc_clk;
end
SEND_UART: begin
adc_clk <= 1'b0;
end
default: begin
adc_clk <= 1'b0;
end
endcase
end
end
// UART state machine
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
tx_busy <= 1'b0;
tx_data <= 8'h00;
tx_count <= 8'h00;
end else begin
if (state == SEND_UART) begin
if (!tx_busy) begin
// send ADC data over UART
case (tx_count)
8'h00: begin
tx_data <= adc_data;
end
8'h01: begin
tx_data <= '\r';
end
8'h02: begin
tx_data <= '\n';
end
default: begin
tx_data <= 8'h00;
end
endcase
if (tx_count == 8'h03) begin
tx_count <= 8'h00;
tx_busy <= 1'b0;
end else begin
tx_count <= tx_count + 1;
tx_busy <= 1'b1;
end
end else begin
// wait for previous byte to finish transmitting
tx_busy <= 1'b1;
end
end else begin
tx_busy <= 1'b0;
tx_data <= 8'h00;
tx_count <= 8'h00;
end
end
end
// UART transmitter
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
// do nothing
end else begin
if (tx_busy) begin
$display("UART: %c", tx_data);
end
end
end
// Output ADC result
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
adc_result <= 8'h00;
end else begin
if (state == READ_ADC) begin
adc_result <= adc_data;
end else begin
adc_result <= 8'h00;
end
end
end
endmodule
```
该模块通过I2C总线控制PCF8591进行ADC转换,并将结果通过UART串口发送到电脑上显示。该模块具有一个状态机,以确定何时启动ADC转换,何时读取ADC结果以及何时发送UART数据。I2C总线和UART串口的控制信号由单独的状态机处理。输出结果为一个8位数字量。
阅读全文
相关推荐
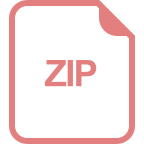


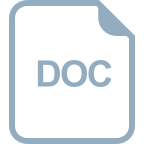






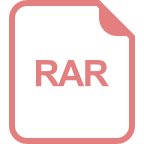
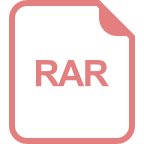



