使用其他的文件读取方法是什么
时间: 2023-12-02 07:04:50 浏览: 28
除了使用标准 C 函数 `fopen_s` 以外,还有其他一些文件读取方法可以尝试,例如:
1. 使用 C++ STL 库中的 ifstream 类:该类提供了更加简洁的文件读取方法,可以方便地读取文件内容。例如:
```C++
#include <fstream>
#include <string>
#include <iostream>
int main() {
std::string filename = "test.txt";
std::ifstream file(filename);
if (!file.is_open()) {
std::cerr << "Error: cannot open file " << filename << std::endl;
return 1;
}
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
return 0;
}
```
2. 使用系统调用函数 open、read:该方法可以使用系统调用来直接读取文件内容,效率较高。例如:
```C++
#include <unistd.h>
#include <fcntl.h>
#include <stdio.h>
int main() {
char buf[1024];
ssize_t nread;
int fd = open("test.txt", O_RDONLY);
if (fd == -1) {
perror("open");
return 1;
}
while ((nread = read(fd, buf, sizeof(buf))) > 0) {
printf("%.*s", (int)nread, buf);
}
close(fd);
return 0;
}
```
需要注意的是,使用系统调用函数需要对文件描述符和错误处理有一定的了解。
以上是两种常见的文件读取方法,您可以根据自己的需求选择适合的方法。
相关推荐
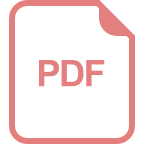
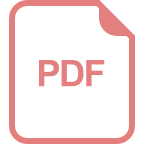
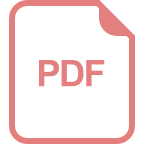
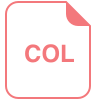
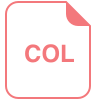
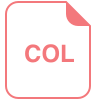
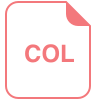
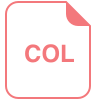










