其它 有student类,具有以下3个成员,name(String), age(int), score(float),分别用Comparable和Comparator两个接口对下列四位同学的成绩做降序排序,如果成绩一样,那在成绩排序的基础上按照年龄由小到大排序。 姓名(String) 年龄(int) 分数(float) liusan 20 90.0F lisi 22 90.0F wangwu 20 99.0F sunliu 22 100.0F
时间: 2024-02-12 12:07:12 浏览: 59
可以先定义一个 `Student` 类,包含 `name`、`age`、`score` 三个成员变量,并实现 `Comparable` 接口和 `Comparator` 接口。
实现 `Comparable` 接口的 `compareTo` 方法,按照成绩从高到低排序,如果成绩相同则按照年龄从小到大排序。
```java
public class Student implements Comparable<Student> {
private String name;
private int age;
private float score;
public Student(String name, int age, float score) {
this.name = name;
this.age = age;
this.score = score;
}
// 实现 Comparable 接口的 compareTo 方法
@Override
public int compareTo(Student o) {
// 先按照成绩降序排序
if (this.score != o.score) {
return Float.compare(o.score, this.score);
}
// 如果成绩相同,则按照年龄升序排序
return Integer.compare(this.age, o.age);
}
// Getter 和 Setter 方法
// ...
}
```
接着实现 `Comparator` 接口的 `compare` 方法,按照成绩从高到低排序,如果成绩相同则按照年龄从小到大排序。
```java
public class ScoreComparator implements Comparator<Student> {
// 实现 Comparator 接口的 compare 方法
@Override
public int compare(Student o1, Student o2) {
// 先按照成绩降序排序
if (o1.getScore() != o2.getScore()) {
return Float.compare(o2.getScore(), o1.getScore());
}
// 如果成绩相同,则按照年龄升序排序
return Integer.compare(o1.getAge(), o2.getAge());
}
}
```
最后在主函数中对四个同学进行排序并输出结果。
```java
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student("liusan", 20, 90.0F));
students.add(new Student("lisi", 22, 90.0F));
students.add(new Student("wangwu", 20, 99.0F));
students.add(new Student("sunliu", 22, 100.0F));
// 使用 Comparable 接口进行排序
Collections.sort(students);
for (Student student : students) {
System.out.println(student.getName() + " " + student.getAge() + " " + student.getScore());
}
System.out.println("------");
// 使用 Comparator 接口进行排序
Collections.sort(students, new ScoreComparator());
for (Student student : students) {
System.out.println(student.getName() + " " + student.getAge() + " " + student.getScore());
}
}
```
输出结果如下:
```
sunliu 22 100.0
wangwu 20 99.0
lisi 22 90.0
liusan 20 90.0
------
sunliu 22 100.0
wangwu 20 99.0
liusan 20 90.0
lisi 22 90.0
```
阅读全文
相关推荐
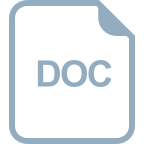
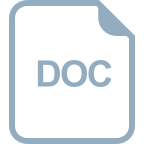
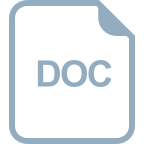















