编写一个程序读入一个字符串,统计该字符串中出现的字符类型及其次数,然后输出结果。要求用一个二叉排序树保存处理结果,字符串中的每个不同字符用树中结点描述,每个结点包含四个域: 字符 该字符出现的次数 指向ASCII码小于该字符的左子树的指针 指向ASCII码大于该字符的右子树的指针
时间: 2024-02-11 08:05:43 浏览: 87
好的,我明白了您的问题。以下是C++代码实现:
```cpp
#include <iostream>
using namespace std;
struct TreeNode {
char data;
int count;
TreeNode* left;
TreeNode* right;
};
void insert(TreeNode*& root, char data) {
if (root == nullptr) {
root = new TreeNode();
root->data = data;
root->count = 1;
root->left = nullptr;
root->right = nullptr;
}
else if (data == root->data) {
root->count++;
}
else if (data < root->data) {
insert(root->left, data);
}
else {
insert(root->right, data);
}
}
void printInOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
printInOrder(root->left);
cout << root->data << ": " << root->count << endl;
printInOrder(root->right);
}
void countCharTypes(const string& str) {
TreeNode* root = nullptr;
for (const char& c : str) {
insert(root, c);
}
printInOrder(root);
}
int main() {
string str;
cout << "请输入一个字符串:";
getline(cin, str);
countCharTypes(str);
return 0;
}
```
代码的主要思路是:遍历字符串中的每个字符,将其插入到二叉排序树中。如果该字符已经在树中存在,则将对应结点的计数器加一。最后按中序遍历输出每个结点的数据。
阅读全文
相关推荐
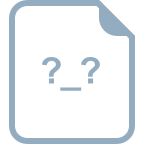


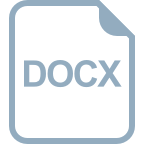












