python中mediapipe如何识别嘴巴
时间: 2023-05-15 15:03:23 浏览: 562
在Python中使用Mediapipe识别嘴巴可以通过以下步骤:
1. 导入必要的库:首先需要导入Mediapipe和OpenCV库,安装完这两个库之后就可以在代码中使用。
2. 载入嘴部标记:下载口部标记,并使用Mediapipe加载该标记。嘴部标记是Mediapipe用于检测嘴部的数据集。
3. 获取嘴部关键点:使用Mediapipe模型检测面部,并获取嘴部的21个关键点。这些关键点包括嘴唇,牙齿和舌头等。
4. 计算嘴部特征:根据21个嘴部关键点的位置,可以计算嘴部形变,包括嘴巴的宽度,高度和曲率等。这些特征可以用于识别不同的表情。
5. 绘制嘴部轮廓:使用OpenCV库将嘴部形变显示在摄像头的图像中,这样就可以实时看到嘴部的变化和不同表情的效果。
总的来说,使用Mediapipe识别嘴巴并检测其形变,是比较复杂的任务。因为嘴部的形态和表情较为多样,所以需要进行大量的数据准备和模型训练。但是,通过使用Mediapipe这样的先进技术,可以大大简化嘴部识别这个任务。同时,使用Mediapipe开发嘴部检测应用,并将其在移动端或者原型机上使用,是涉及虚拟场景、多媒体、人机交互等多个领域的一项重要技术。”
相关问题
使用MediaPipe实现摄像头手、嘴巴和眼睛识别分割的示例代码
以下是使用MediaPipe实现摄像头手、嘴巴和眼睛识别分割的示例代码:
```python
import cv2
import mediapipe as mp
# 初始化MediaPipe手、嘴巴和眼睛模型
mp_hands = mp.solutions.hands
mp_face_mesh = mp.solutions.face_mesh
# 初始化摄像头
cap = cv2.VideoCapture(0)
while cap.isOpened():
# 读取摄像头图像
success, image = cap.read()
if not success:
continue
# 对图像进行预处理
image = cv2.cvtColor(cv2.flip(image, 1), cv2.COLOR_BGR2RGB)
image.flags.writeable = False
# 运行手、嘴巴和眼睛模型
with mp_hands.Hands(min_detection_confidence=0.5, min_tracking_confidence=0.5) as hands:
results = hands.process(image)
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
# 处理手部关键点
# ...
with mp_face_mesh.FaceMesh(min_detection_confidence=0.5, min_tracking_confidence=0.5) as face_mesh:
results = face_mesh.process(image)
if results.multi_face_landmarks:
for face_landmarks in results.multi_face_landmarks:
# 处理面部关键点
# ...
# 将手、嘴巴和眼睛的关键点进行合并
landmarks = []
if results.multi_hand_landmarks:
for hand_landmarks in results.multi_hand_landmarks:
for landmark in hand_landmarks.landmark:
landmarks.append((landmark.x, landmark.y, landmark.z))
if results.multi_face_landmarks:
for face_landmarks in results.multi_face_landmarks:
for landmark in face_landmarks.landmark:
landmarks.append((landmark.x, landmark.y, landmark.z))
# 在图像中绘制关键点
for landmark in landmarks:
x, y = int(landmark[0] * image.shape[1]), int(landmark[1] * image.shape[0])
cv2.circle(image, (x, y), 5, (0, 255, 0), -1)
# 显示处理结果
cv2.imshow('MediaPipe Hands and FaceMesh', image)
if cv2.waitKey(5) & 0xFF == 27:
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
在上述示例代码中,我们首先初始化了MediaPipe的手、嘴巴和眼睛模型,并使用摄像头读取图像。随后,我们对图像进行了预处理,将图像转换为RGB格式,并禁用了图像的写入功能,以便更快地处理图像。然后,我们分别使用MediaPipe的手和面部模型处理图像,并将手、嘴巴和眼睛的关键点进行合并。最后,我们在图像中绘制关键点,并将处理结果显示在窗口中。请注意,上述示例代码仅用于演示MediaPipe实现摄像头手、嘴巴和眼睛识别分割的基本原理,具体的实现细节需要根据您的需求进行调整。
mediapipe实现手部、嘴巴和眼睛的分割代码
以下是使用MediaPipe实现手部、嘴巴和眼睛分割的示例代码:
``` python
import cv2
import mediapipe as mp
mp_drawing = mp.solutions.drawing_utils
mp_hands = mp.solutions.hands
mp_face_mesh = mp.solutions.face_mesh
mp_face_detection = mp.solutions.face_detection
# 初始化Hand和Face Mesh模型
hands = mp_hands.Hands()
face_mesh = mp_face_mesh.FaceMesh()
face_detection = mp_face_detection.FaceDetection()
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
success, image = cap.read()
if not success:
print("Could not read from camera.")
break
# 调整图像的大小和颜色空间
image = cv2.cvtColor(cv2.flip(image, 1), cv2.COLOR_BGR2RGB)
image.flags.writeable = False
# 检测手部关键点
hands_results = hands.process(image)
if hands_results.multi_hand_landmarks:
for hand_landmarks in hands_results.multi_hand_landmarks:
mp_drawing.draw_landmarks(image, hand_landmarks, mp_hands.HAND_CONNECTIONS)
# 检测面部关键点
face_mesh_results = face_mesh.process(image)
if face_mesh_results.multi_face_landmarks:
for face_landmarks in face_mesh_results.multi_face_landmarks:
mp_drawing.draw_landmarks(image, face_landmarks, mp_face_mesh.FACE_CONNECTIONS)
# 检测面部特征点
face_detection_results = face_detection.process(image)
if face_detection_results.detections:
for detection in face_detection_results.detections:
mp_drawing.draw_detection(image, detection)
# 显示图像
cv2.imshow('MediaPipe Hands and Face Mesh', cv2.cvtColor(image, cv2.COLOR_RGB2BGR))
if cv2.waitKey(5) & 0xFF == 27:
break
# 释放资源
hands.close()
face_mesh.close()
face_detection.close()
cap.release()
cv2.destroyAllWindows()
```
这段代码使用了MediaPipe提供的Hands、Face Mesh和Face Detection模型,分别检测手部关键点、面部关键点和面部特征点,并在图像上绘制出检测结果。您可以根据需要修改代码,以实现更多的功能。
阅读全文
相关推荐
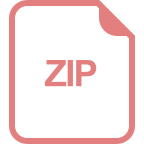
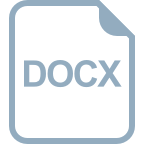
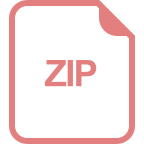










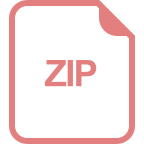
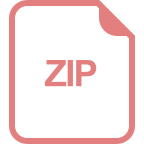
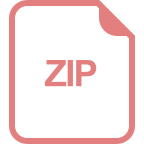
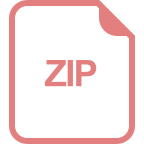