#include <iostream> #include <vector> #include <algorithm> #include <climits> using namespace std; int getMinTransDelay(int n, vector<vector<int>>& times, int src, int tar) { vector<vector<pair<int, int>>> graph(n + 1); // 构建图 for (auto& time : times) { int u = time[0], v = time[1], w = time[2]; graph[u].push_back({v, w}); } // 初始化距离数组 vector<int> dist(n + 1, INT_MAX); dist[src] = 0; // 初始化待检查节点数组 vector<int> toCheck; toCheck.push_back(src); while (!toCheck.empty()) { bool flag = false; int u = toCheck[0]; // 遍历相邻节点 for (auto& next : graph[u]) { int v = next.first, w = next.second; int newDist = dist[u] + w; // 更新最短距离 if (newDist >= dist[v]) continue; dist[v] = newDist; // 将新节点加入待检查数组 if (find(toCheck.begin(), toCheck.end(), v) == toCheck.end()) { toCheck.push_back(v); flag = true; } } // 移除当前节点并按距离排序 if (!flag) toCheck.erase(toCheck.begin()); else sort(toCheck.begin(), toCheck.end(), [&](int a, int b) { return dist[a] < dist[b]; }); } return dist[tar] == INT_MAX ? -1 : dist[tar]; } int main() { int n, m; vector<vector<int>> times; int src, tar; // 读入数据 cin >> n >> m; for (int i = 0; i < m; i++) { int u, v, w; cin >> u >> v >> w; times.push_back({u, v, w}); } cin >> src >> tar; // 计算最短传输时延 cout << getMinTransDelay(n, times, src, tar) << endl; return 0; } 这段代码用Python怎么写
时间: 2024-04-16 12:24:56 浏览: 167
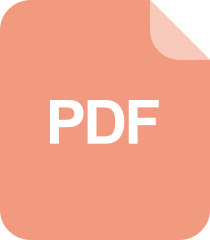
2048C++实现代码
以下是将上述 C++ 代码转换为 Python 代码的示例:
```python
import sys
def getMinTransDelay(n, times, src, tar):
graph = [[] for _ in range(n + 1)]
# 构建图
for time in times:
u, v, w = time[0], time[1], time[2]
graph[u].append((v, w))
# 初始化距离数组
dist = [float('inf')] * (n + 1)
dist[src] = 0
# 初始化待检查节点数组
toCheck = [src]
while toCheck:
flag = False
u = toCheck[0]
# 遍历相邻节点
for next in graph[u]:
v, w = next[0], next[1]
newDist = dist[u] + w
# 更新最短距离
if newDist >= dist[v]:
continue
dist[v] = newDist
# 将新节点加入待检查数组
if v not in toCheck:
toCheck.append(v)
flag = True
# 移除当前节点并按距离排序
if not flag:
toCheck.pop(0)
else:
toCheck.sort(key=lambda x: dist[x])
return dist[tar] if dist[tar] != float('inf') else -1
def main():
n, m = map(int, input().split())
times = []
for _ in range(m):
u, v, w = map(int, input().split())
times.append([u, v, w])
src, tar = map(int, input().split())
# 计算最短传输时延
print(getMinTransDelay(n, times, src, tar))
if __name__ == "__main__":
main()
```
请注意,Python 的语法和一些库函数与 C++ 有所不同,因此在进行转换时需要逐行进行调整。此示例代码应与原始 C++ 代码功能相同。
希望对你有所帮助!如有任何疑问,请随时提问。
阅读全文
相关推荐
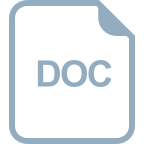
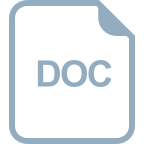
















