用c++和贪心算法解决这个问题:描述 We assume that there is a turn-based RPG game. You control an undefeated hero. In each round of this game, you can choose one enemy to attack, and its HP will decrease by 1. However, at the same time, all living enemies will attack you, and your HP will decrease by the sum of their DPS. If one enemy's HP drops equal to or below zero, it will die after this round, and it cannot attack you in the following rounds. Although your hero is undefeated, you need to choose the best strategy to kill all the enemies with the minimum HP loss. 输入 There are mutiple test cases. The first line of each test case contains the number of enemies N (1<=N<=20). Then N lines follow, with each containing two integers DPSi and HPi, which are the DPS and HP for each enemy (1<=DPSi, HPi<=1000) respectively. The inputs terminate at the end of the file (EOF). 输出 Output one line for each test, indicating the minimum HP loss.
时间: 2024-02-14 15:04:39 浏览: 131
这个问题也可以使用贪心算法来解决。我们可以把所有敌人按照 DPS 从小到大排序,然后每次选择 DPS 最小的敌人进行攻击,直到所有敌人都被消灭为止。具体实现可以参考下面的 C++ 代码:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
const int MAXN = 20 + 5;
int n, hero_hp;
int dps[MAXN], hp[MAXN];
bool cmp(int a, int b) {
return dps[a] < dps[b];
}
int main() {
while (cin >> n) {
for (int i = 1; i <= n; i++) {
cin >> dps[i] >> hp[i];
}
cin >> hero_hp;
int min_hp_loss = 0;
int alive[MAXN];
for (int i = 1; i <= n; i++) {
alive[i] = 1;
}
sort(alive+1, alive+n+1, cmp);
for (int i = 1; i <= n; i++) {
int j;
for (j = 1; j <= n && hp[alive[j]] <= 0; j++) {
}
if (j > n) {
break;
}
min_hp_loss += dps[alive[j]];
for (int k = 1; k <= n; k++) {
if (alive[k] && hp[alive[k]] > 0) {
hp[alive[k]] -= dps[alive[j]];
}
}
}
cout << min_hp_loss << endl;
}
return 0;
}
```
注意,我们使用了一个数组 alive 来表示每个敌人是否还活着,这是为了方便排序和查找 DPS 最小的敌人。在每次攻击后,我们需要更新所有还活着的敌人的 HP 值。最终的答案即为所有攻击造成的健康值损失之和。
阅读全文
相关推荐




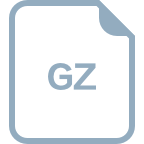
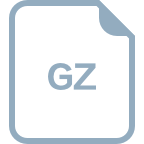
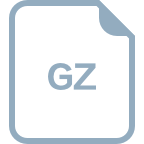








