基于链式存储结构的图书信息表的创建和输出,链表的输入
时间: 2024-09-27 16:16:02 浏览: 43
链式存储结构的图书信息表通常是一个节点数据结构,每个节点包含书的信息(如书名、作者、ISBN等),以及指向下一个节点的指针。创建这样一个表的步骤可以分为以下几个部分:
1. 定义图书节点类(BookNode):
```java
class BookNode {
String title;
String author;
String isbn;
BookNode next; // 指向下一个节点的引用
// 构造函数
public BookNode(String title, String author, String isbn) {
this.title = title;
this.author = author;
this.isbn = isbn;
this.next = null;
}
}
```
2. 创建链表并插入节点:
```java
public class BookList {
private BookNode head;
public void addBook(String title, String author, String isbn) {
if (head == null) {
head = new BookNode(title, author, isbn);
} else {
BookNode newNode = new BookNode(title, author, isbn);
newNode.next = head;
head = newNode;
}
}
// 输出链表中的所有书籍信息
public void printBooks() {
BookNode current = head;
while (current != null) {
System.out.println("Title: " + current.title + ", Author: " + current.author + ", ISBN: " + current.isbn);
current = current.next;
}
}
}
```
3. 输入图书信息:
```java
public static void main(String[] args) {
BookList bookList = new BookList();
bookList.addBook("书名1", "作者1", "ISBN1");
bookList.addBook("书名2", "作者2", "ISBN2");
bookList.printBooks(); // 打印链表中的所有书籍信息
}
```
阅读全文
相关推荐
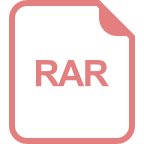
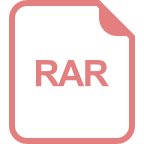
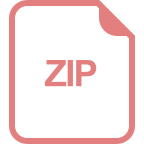















