实现一个简单的计算器,前面是一个文本框(操作数1),后面是标签(操作符),再后面是一个文本框(操作数2),然后是一个"="按钮(JButton),最后是一个文本框(结果) (1)实现按钮ActionEvent事件的处理,点击等号获取计算结果,并显示在最后一个文本框; (2)实现第二个文本框的ActionEvent事件处理,在第二个文本框按回车,直接获得计算结果,并显示在最后一个文本框。(事件源为第二个文本框) (3)实现运算符标签的MouseEvent事件的处理,点击标签,可以更换标签的运算符,比如:将加号改成减号,从而实现减法功能,点击等号按钮,实现减法运算,并将结果显示在第三个文本框中。(具体实现:实现mouseClicked()时,判断鼠标单击次数是否为除4余0、余1、余2、余3,比如余为1,则将运算符标签上的字符修改为”-”,从而实现减法运算)
时间: 2024-02-23 21:03:00 浏览: 59
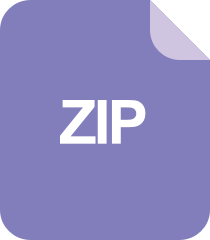
简单的计算器程序,可以使用按钮+键盘两种方式输入

以下是一个简单的实现:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Calculator extends JFrame implements ActionListener, KeyListener, MouseListener {
private JTextField operand1Field, operand2Field, resultField;
private JLabel operatorLabel;
private JButton equalsButton;
public Calculator() {
super("Calculator");
// Create GUI components
operand1Field = new JTextField(10);
operand2Field = new JTextField(10);
resultField = new JTextField(10);
resultField.setEditable(false);
operatorLabel = new JLabel("+");
operatorLabel.addMouseListener(this);
equalsButton = new JButton("=");
equalsButton.addActionListener(this);
// Add components to content pane
Container c = getContentPane();
c.setLayout(new FlowLayout());
c.add(operand1Field);
c.add(operatorLabel);
c.add(operand2Field);
c.add(equalsButton);
c.add(resultField);
// Add key listeners to operand fields
operand1Field.addKeyListener(this);
operand2Field.addKeyListener(this);
// Set window properties and display
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(300, 100);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == equalsButton) {
calculate();
}
}
public void keyPressed(KeyEvent e) {
if (e.getSource() == operand1Field || e.getSource() == operand2Field) {
if (e.getKeyCode() == KeyEvent.VK_ENTER) {
calculate();
}
}
}
public void mouseClicked(MouseEvent e) {
if (e.getSource() == operatorLabel) {
int clicks = e.getClickCount();
if (clicks % 4 == 1) {
operatorLabel.setText("-");
} else if (clicks % 4 == 2) {
operatorLabel.setText("*");
} else if (clicks % 4 == 3) {
operatorLabel.setText("/");
} else {
operatorLabel.setText("+");
}
}
}
public void calculate() {
try {
double operand1 = Double.parseDouble(operand1Field.getText());
double operand2 = Double.parseDouble(operand2Field.getText());
char operator = operatorLabel.getText().charAt(0);
double result;
switch (operator) {
case '+':
result = operand1 + operand2;
break;
case '-':
result = operand1 - operand2;
break;
case '*':
result = operand1 * operand2;
break;
case '/':
result = operand1 / operand2;
break;
default:
throw new IllegalArgumentException("Invalid operator: " + operator);
}
resultField.setText(Double.toString(result));
} catch (NumberFormatException ex) {
resultField.setText("Error");
}
}
// Unused event listener methods
public void keyTyped(KeyEvent e) {}
public void keyReleased(KeyEvent e) {}
public void mousePressed(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
public static void main(String[] args) {
Calculator calc = new Calculator();
}
}
```
这个实现中,`Calculator` 类继承了 `JFrame` 类,实现了 `ActionListener`、`KeyListener` 和 `MouseListener` 接口。在构造函数中,先创建了各个 GUI 组件,然后把它们添加到内容面板中。并为操作数文本框添加了键盘事件监听器。在 `actionPerformed()` 方法中,当用户按下“=”按钮时,调用 `calculate()` 方法进行计算。在 `keyPressed()` 方法中,当用户在操作数文本框中按下回车键时,也调用 `calculate()` 方法。在 `mouseClicked()` 方法中,当用户单击运算符标签时,根据单击次数修改运算符标签上的字符。`calculate()` 方法中,解析操作数文本框中的文本,解析运算符标签上的字符,进行计算,然后将结果显示在结果文本框中。
阅读全文
相关推荐
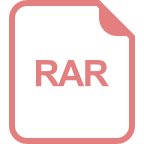














