请帮我用C++与Opencv实现,输入一个像素坐标点,得到其周围4领域像素点的颜色值
时间: 2024-03-01 22:50:22 浏览: 31
好的,你可以使用OpenCV中的cv::Mat对象来读取和处理像素值。下面是一个简单的示例代码,可以实现输入一个像素坐标点,得到其周围4领域像素点的颜色值:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(){
Mat img = imread("image.jpg", IMREAD_COLOR); // 读取图片
if (img.empty()) {
cout << "Can't read image file." << endl;
return -1;
}
Point pt(100, 100); // 设置像素坐标点
Vec3b color = img.at<Vec3b>(pt); // 获取像素点的颜色值
// 获取周围4领域点的颜色值
Point left(pt.x-1, pt.y);
Point right(pt.x+1, pt.y);
Point up(pt.x, pt.y-1);
Point down(pt.x, pt.y+1);
Vec3b left_color = img.at<Vec3b>(left);
Vec3b right_color = img.at<Vec3b>(right);
Vec3b up_color = img.at<Vec3b>(up);
Vec3b down_color = img.at<Vec3b>(down);
// 输出颜色值
cout << "Color at (" << pt.x << ", " << pt.y << "): " << color << endl;
cout << "Color at left point: " << left_color << endl;
cout << "Color at right point: " << right_color << endl;
cout << "Color at up point: " << up_color << endl;
cout << "Color at down point: " << down_color << endl;
return 0;
}
```
在这个示例中,我们读取了一张图片,并且定义了一个像素坐标点pt。然后使用img.at<Vec3b>(pt)函数获取了该像素点的颜色值,使用类似的方法获取周围4领域像素点的颜色值。最后,我们将颜色值输出到控制台上。
需要注意的是,这个示例只是一个简单的实现方式,实际应用中需要根据具体需求进行调整和优化。
相关推荐
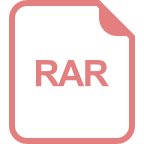














