输入一行字符,分别统计出其中英文字母,数字和其他字符的个数,用 c++实现
时间: 2024-10-18 14:22:02 浏览: 29
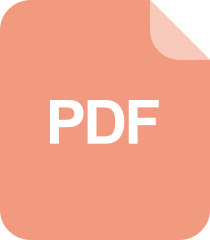
C++统计中英文大小写字母、数字、空格及其他字符个数的方法

在 C++ 中,你可以使用标准库中的 `<cctype>` 和 `<clocale>` 来统计输入字符串中字母、数字和其他字符的数量。以下是一个简单的示例:
```cpp
#include <iostream>
#include <cctype>
#include <locale>
int count_chars(std::string input) {
int alpha_count = 0;
int digit_count = 0;
int other_count = 0;
// 创建本地化对象,以便正确处理不同语言环境下的字符分类
std::locale loc("");
for (char c : input) {
if (std::isalpha(c, loc)) { // 检查是否为字母
alpha_count++;
} else if (std::isdigit(c, loc)) { // 检查是否为数字
digit_count++;
} else { // 其他字符
other_count++;
}
}
return std::make_tuple(alpha_count, digit_count, other_count);
}
int main() {
std::string user_input;
std::cout << "请输入一行字符:";
std::getline(std::cin, user_input);
std::tuple<int, int, int> counts = count_chars(user_input);
std::cout << "英文字母数量:\t\t" << get<0>(counts) << '\n';
std::cout << "数字数量:\t\t" << get<1>(counts) << '\n';
std::cout << "其他字符数量:\t" << get<2>(counts) << '\n';
return 0;
}
```
这个程序首先获取用户输入,然后遍历每个字符并使用相应的 C 标准库函数 (`std::isalpha` 和 `std::isdigit`) 来判断其类别。最后,它会返回一个包含三个元素的元组,分别表示字母、数字和其它字符的数量。
阅读全文
相关推荐
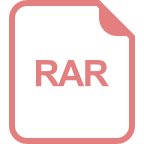
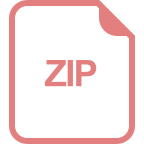















