qt opencv获取像素点的像素值
时间: 2023-08-30 09:02:29 浏览: 104
在Qt中使用OpenCV获取像素点的像素值步骤如下:
首先,使用OpenCV加载图片,可以使用cv::imread函数来完成,将图片路径作为参数传入该函数中。例如,可以使用以下代码加载一张名为"image.png"的图片:
cv::Mat image = cv::imread("image.png");
接下来,我们可以使用OpenCV中的at函数来获取指定像素点的像素值。at函数的参数是像素点的行列索引。通常情况下,OpenCV中的图像是以BGR格式存储的,即每个像素点有三个通道,分别代表蓝色、绿色和红色。我们可以通过at函数来获取每个通道的像素值,然后组合起来。
以下是示例代码,用于获取图像中第10行第20列像素点的像素值:
int blue = image.at<cv::Vec3b>(10, 20)[0];
int green = image.at<cv::Vec3b>(10, 20)[1];
int red = image.at<cv::Vec3b>(10, 20)[2];
注意,返回的像素值是以整数形式表示的。
通过这样的步骤,我们可以在Qt中使用OpenCV获取特定像素点的像素值。
相关问题
qt中opencv怎么获取像素点灰度分布并绘制直方图
以下是在Qt中使用OpenCV获取像素点灰度分布并绘制直方图的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <QImage>
#include <QPixmap>
#include <QLabel>
#include <QVBoxLayout>
#include <QHBoxLayout>
#include <QFileDialog>
#include <QDebug>
using namespace cv;
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QWidget widget;
QVBoxLayout *layout = new QVBoxLayout(&widget);
// 选择图片
QString fileName = QFileDialog::getOpenFileName(&widget, "Open Image", ".", "Image Files (*.png *.jpg *.bmp)");
if (fileName.isNull()) {
return 0;
}
// 读取图片并转换为灰度图
Mat image = imread(fileName.toStdString());
cvtColor(image, image, CV_BGR2GRAY);
// 计算像素点灰度分布
int histSize = 256;
float range[] = { 0, 256 };
const float* histRange = { range };
bool uniform = true;
bool accumulate = false;
Mat hist;
calcHist(&image, 1, 0, Mat(), hist, 1, &histSize, &histRange, uniform, accumulate);
// 绘制直方图
int histWidth = 512;
int histHeight = 400;
int binWidth = cvRound((double)histWidth / histSize);
Mat histImage(histHeight, histWidth, CV_8UC1, Scalar(255, 255, 255));
normalize(hist, hist, 0, histImage.rows, NORM_MINMAX, -1, Mat());
for (int i = 1; i < histSize; i++) {
line(histImage, Point(binWidth * (i - 1), histHeight - cvRound(hist.at<float>(i - 1))),
Point(binWidth * i, histHeight - cvRound(hist.at<float>(i))),
Scalar(0, 0, 0), 2, LINE_AA);
}
// 将结果显示在界面上
QLabel *imageLabel = new QLabel(&widget);
QImage qImage(image.data, image.cols, image.rows, QImage::Format_Grayscale8);
QPixmap pixmap = QPixmap::fromImage(qImage);
imageLabel->setPixmap(pixmap);
QLabel *histLabel = new QLabel(&widget);
QImage qHistImage(histImage.data, histImage.cols, histImage.rows, QImage::Format_Grayscale8);
QPixmap histPixmap = QPixmap::fromImage(qHistImage);
histLabel->setPixmap(histPixmap);
QHBoxLayout *hLayout = new QHBoxLayout();
hLayout->addWidget(imageLabel);
hLayout->addWidget(histLabel);
layout->addLayout(hLayout);
widget.show();
return a.exec();
}
```
该代码首先通过 `cv::imread()` 函数读取指定的图片,并将其转换为灰度图。然后使用 `cv::calcHist()` 函数计算像素点灰度分布。
接下来,代码创建一个 `cv::Mat` 对象来绘制直方图。在这个对象中,每个像素代表一个灰度值的计数。通过 `cv::normalize()` 函数将这些计数转换为像素点的数量,以便在绘制直方图时进行比例缩放。最后,使用 `cv::line()` 函数在 `cv::Mat` 对象上绘制直方图。
最后,代码将原始图片和直方图分别显示在 `QLabel` 控件中,然后将这些控件放置在 `QHBoxLayout` 容器中,并将该容器添加到 `QVBoxLayout` 容器中。最终,通过调用 `QWidget::show()` 函数显示主窗口。
qt中opencv怎么将某个灰度值以下的像素点设为黑色
可以使用cv::threshold函数来实现将某个灰度值以下的像素点设为黑色。具体步骤如下:
1. 将图像转换为灰度图像。
2. 使用cv::threshold函数进行阈值处理,将灰度值小于设定值的像素点设为黑色,大于等于设定值的像素点设为白色。
示例代码如下:
```
cv::Mat grayImage;
cv::cvtColor(image, grayImage, cv::COLOR_BGR2GRAY); // 将图像转换为灰度图像
int thresholdValue = 128; // 设定阈值为128
cv::Mat binaryImage;
cv::threshold(grayImage, binaryImage, thresholdValue, 255, cv::THRESH_BINARY); // 阈值处理
cv::imshow("Original Image", image);
cv::imshow("Binary Image", binaryImage);
cv::waitKey(0);
```
上述代码将图像转换为灰度图像后,设定阈值为128,使用cv::threshold函数进行阈值处理,将灰度值小于128的像素点设为黑色,大于等于128的像素点设为白色。最后显示原始图像和处理后的二值图像。
相关推荐
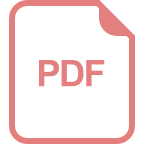
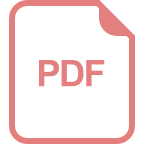












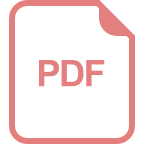