stm32f103c8t6模拟i2c代码
时间: 2024-04-07 14:26:32 浏览: 90
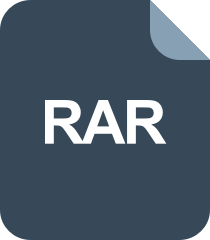
STM32F103C8T6使用HAL库创建0.96‘OLED模拟I2C显示例程
STM32F103C8T6是一款常用的单片机,支持模拟I2C通信。下面是一个简单的示例代码,用于在STM32F103C8T6上实现模拟I2C通信:
```c
#include "stm32f10x.h"
#define I2C_SCL_PIN GPIO_Pin_6
#define I2C_SDA_PIN GPIO_Pin_7
#define I2C_GPIO_PORT GPIOB
void I2C_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = I2C_SCL_PIN | I2C_SDA_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_Init(I2C_GPIO_PORT, &GPIO_InitStructure);
GPIO_SetBits(I2C_GPIO_PORT, I2C_SCL_PIN | I2C_SDA_PIN);
}
void I2C_Start(void)
{
GPIO_SetBits(I2C_GPIO_PORT, I2C_SCL_PIN | I2C_SDA_PIN);
GPIO_ResetBits(I2C_GPIO_PORT, I2C_SDA_PIN);
GPIO_ResetBits(I2C_GPIO_PORT, I2C_SCL_PIN);
}
void I2C_Stop(void)
{
GPIO_ResetBits(I2C_GPIO_PORT, I2C_SCL_PIN);
GPIO_ResetBits(I2C_GPIO_PORT, I2C_SDA_PIN);
GPIO_SetBits(I2C_GPIO_PORT, I2C_SCL_PIN | I2C_SDA_PIN);
}
void I2C_SendByte(uint8_t byte)
{
uint8_t i;
for (i = 0; i < 8; i++)
{
GPIO_ResetBits(I2C_GPIO_PORT, I2C_SCL_PIN);
if (byte & 0x80)
GPIO_SetBits(I2C_GPIO_PORT, I2C_SDA_PIN);
else
GPIO_ResetBits(I2C_GPIO_PORT, I2C_SDA_PIN);
GPIO_SetBits(I2C_GPIO_PORT, I2C_SCL_PIN);
byte <<= 1;
}
GPIO_ResetBits(I2C_GPIO_PORT, I2C_SCL_PIN);
}
uint8_t I2C_ReceiveByte(void)
{
uint8_t i, byte = 0;
GPIO_SetBits(I2C_GPIO_PORT, I2C_SDA_PIN);
for (i = 0; i < 8; i++)
{
byte <<= 1;
GPIO_ResetBits(I2C_GPIO_PORT, I2C_SCL_PIN);
if (GPIO_ReadInputDataBit(I2C_GPIO_PORT, I2C_SDA_PIN))
byte |= 0x01;
GPIO_SetBits(I2C_GPIO_PORT, I2C_SCL_PIN);
}
GPIO_ResetBits(I2C_GPIO_PORT, I2C_SCL_PIN);
return byte;
}
void I2C_WriteByte(uint8_t addr, uint8_t reg, uint8_t data)
{
I2C_Start();
I2C_SendByte(addr << 1);
I2C_SendByte(reg);
I2C_SendByte(data);
I2C_Stop();
}
uint8_t I2C_ReadByte(uint8_t addr, uint8_t reg)
{
uint8_t data;
I2C_Start();
I2C_SendByte(addr << 1);
I2C_SendByte(reg);
I2C_Start();
I2C_SendByte((addr << 1) | 0x01);
data = I2C_ReceiveByte();
I2C_Stop();
return data;
}
int main(void)
{
I2C_Init();
// 使用示例:向地址为0x50的设备的寄存器0x10写入数据0xAA
I2C_WriteByte(0x50, 0x10, 0xAA);
// 使用示例:从地址为0x50的设备的寄存器0x10读取数据
uint8_t data = I2C_ReadByte(0x50, 0x10);
while (1)
{
// 主循环
}
}
```
这段代码实现了模拟I2C通信的基本功能,包括初始化I2C引脚、发送起始信号、发送字节、接收字节等操作。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
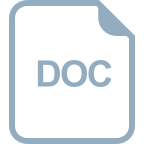
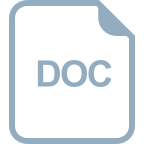
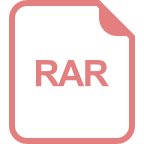
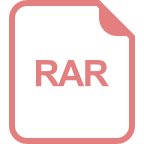
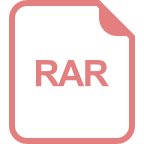
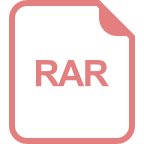
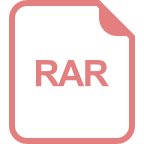
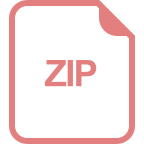
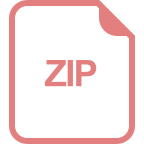
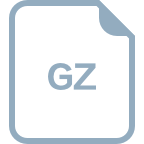
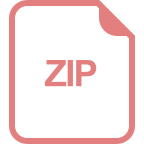
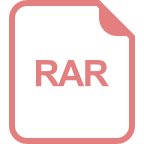
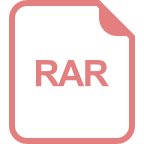
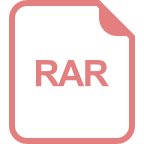



