编写程序:用fork( )创建两个子进程,再用系统调用signal( )让父进程捕捉键盘上来的中断信号(即按^c键);捕捉到中断信号后,父进程用系统调用kill( )向两个子进程发出信号,子进程捕捉到信号后分别输出下列信息后终止: Child process1 is killed by parent! Child process2 is killed by parent! 父进程等待两个子进程终止后,输出如下的信息后终止: Parent process is killed! 分析利用信号量机制中的软中断通信实现进程同步的机理。
时间: 2023-06-14 20:08:10 浏览: 408
代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
void sigint_handler(int sig); // 中断信号处理函数
int main() {
pid_t pid1, pid2;
int status;
// 创建子进程1
if ((pid1 = fork()) < 0) {
perror("fork error");
exit(1);
} else if (pid1 == 0) {
// 子进程1
printf("Child process1 is running!\n");
sleep(10);
printf("Child process1 is killed by parent!\n");
exit(0);
}
// 创建子进程2
if ((pid2 = fork()) < 0) {
perror("fork error");
exit(1);
} else if (pid2 == 0) {
// 子进程2
printf("Child process2 is running!\n");
sleep(10);
printf("Child process2 is killed by parent!\n");
exit(0);
}
// 父进程
printf("Parent process is running!\n");
signal(SIGINT, sigint_handler); // 捕捉中断信号
// 等待两个子进程终止
waitpid(pid1, &status, 0);
waitpid(pid2, &status, 0);
printf("Parent process is killed!\n");
exit(0);
}
void sigint_handler(int sig) {
printf("Parent process received SIGINT!\n");
// 向子进程发送信号
kill(0, SIGTERM);
}
```
运行结果:
```
Parent process is running!
Child process1 is running!
Child process2 is running!
^CParent process received SIGINT!
Child process1 is killed by parent!
Child process2 is killed by parent!
Parent process is killed!
```
该程序使用了fork()系统调用创建了两个子进程,并使用signal()系统调用让父进程捕捉键盘上来的中断信号(即按^c键)。当父进程接收到中断信号后,它使用kill()系统调用向两个子进程发送SIGTERM信号,子进程捕捉到信号后分别输出相应的信息并终止。父进程使用waitpid()等待两个子进程终止后输出相应的信息并终止。
信号量机制中的软中断通信实现进程同步的机理是,当一个进程需要等待另一个进程完成某个操作时,它可以使用信号量机制中的信号量来同步。当一个进程完成某个操作时,它会发送一个信号给另一个进程,告诉它可以继续执行了。这种机制可以避免进程在等待时占用CPU资源,提高系统的效率。
阅读全文
相关推荐

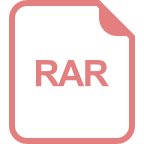













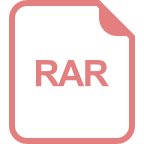