修改#检测目标 detections1 = model(image1) detections2 = model(image2) detections3 = model(image3)报错RuntimeError: expected scalar type Byte but found Float
时间: 2024-01-14 18:04:45 浏览: 20
这个错误通常是由于输入图像的像素值类型不正确导致的。YOLOv7模型要求输入图像像素值为8位无符号整数(即`uint8`类型),而您提供的输入图像像素值可能是浮点数(即`float`类型)。您可以使用以下代码将输入图像像素值类型转换为`uint8`类型:
```
import torch
import numpy as np
# 转换图像为PyTorch张量
image1 = torch.from_numpy(np.ascontiguousarray(image1.astype(np.uint8))).permute(2, 0, 1).unsqueeze(0).to(device)
image2 = torch.from_numpy(np.ascontiguousarray(image2.astype(np.uint8))).permute(2, 0, 1).unsqueeze(0).to(device)
image3 = torch.from_numpy(np.ascontiguousarray(image3.astype(np.uint8))).permute(2, 0, 1).unsqueeze(0).to(device)
# 检测目标
detections1 = model(image1)
detections2 = model(image2)
detections3 = model(image3)
```
这将确保您的输入图像像素值类型正确,并且可以被YOLOv7模型正确处理。
相关问题
修改# 检测目标 detections1 = model(image1) detections2 = model(image2) detections3 = model(image3)报错 Expected 4-dimensional input for 4-dimensional weight [32, 3, 3, 3], but got 3-dimensional input of size [4032, 3024, 3] instead
这个错误是因为YOLOv7模型期望的输入是一个4维张量,而您提供的输入是一个3维张量。您需要将输入转换为4维张量。这可以通过在输入张量的第一维插入一个大小为1的维度来完成。您可以使用以下代码:
```
import torch
import numpy as np
# 转换图像为PyTorch张量
image1 = torch.from_numpy(np.ascontiguousarray(image1)).permute(2, 0, 1).unsqueeze(0).to(device)
image2 = torch.from_numpy(np.ascontiguousarray(image2)).permute(2, 0, 1).unsqueeze(0).to(device)
image3 = torch.from_numpy(np.ascontiguousarray(image3)).permute(2, 0, 1).unsqueeze(0).to(device)
# 检测目标
detections1 = model(image1)
detections2 = model(image2)
detections3 = model(image3)
```
这将确保您的输入张量是一个4维张量,可以传递给YOLOv7模型进行检测。
修改# 对每个相机图像进行目标检测 detections1 = model(image1) # 检测结果1 detections2 = model(image2) # 检测结果2 detections3 = model(image3) # 检测结果3 # 对检测结果进行筛选 filtered_detections1 = non_max_suppression(detections1, confidence_threshold, iou_threshold) filtered_detections2 = non_max_suppression(detections2, confidence_threshold, iou_threshold) filtered_detections3 = non_max_suppression(detections3, confidence_threshold, iou_threshold)报错TypeError: conv2d(): argument 'input' (position 1) must be Tensor, not numpy.ndarray
这个错误是因为YOLOv7输出的是一个numpy数组,而非PyTorch张量,因此无法在PyTorch中进一步处理。您需要将numpy数组转换为PyTorch张量,可以使用以下代码:
```
import torch
import numpy as np
# 转换图像为PyTorch张量
image1 = torch.from_numpy(np.ascontiguousarray(image1)).to(device)
image2 = torch.from_numpy(np.ascontiguousarray(image2)).to(device)
image3 = torch.from_numpy(np.ascontiguousarray(image3)).to(device)
# 检测目标
detections1 = model(image1)
detections2 = model(image2)
detections3 = model(image3)
# 转换检测结果为PyTorch张量
detections1 = [torch.from_numpy(x) for x in detections1]
detections2 = [torch.from_numpy(x) for x in detections2]
detections3 = [torch.from_numpy(x) for x in detections3]
# 对检测结果进行筛选
filtered_detections1 = non_max_suppression(detections1, confidence_threshold, iou_threshold)
filtered_detections2 = non_max_suppression(detections2, confidence_threshold, iou_threshold)
filtered_detections3 = non_max_suppression(detections3, confidence_threshold, iou_threshold)
```
这将确保您的检测结果可以在PyTorch中进一步处理。
相关推荐
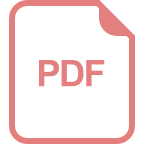











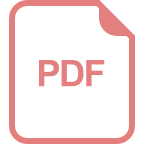
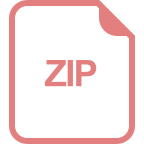