matlab实现:C是空间城市三维坐标,D是距离矩阵,遗传算法解决TSP问题,要求迭代绘制每一次的最优路径,注意坐标是三维的
时间: 2024-04-03 12:32:16 浏览: 75
好的,以下是基于遗传算法解决TSP问题的MATLAB实现,可以迭代绘制每一次的最优路径:
```matlab
% 遗传算法解决TSP问题
% 城市坐标
C = [x1,y1,z1;x2,y2,z2;...;xn,yn,zn];
% 距离矩阵
D = pdist2(C,C);
% 参数设置
popsize = 100; % 种群大小
pc = 0.8; % 交叉概率
pm = 0.2; % 变异概率
maxgen = 100; % 最大迭代次数
% 初始化种群
pop = zeros(popsize,n);
for i = 1:popsize
pop(i,:) = randperm(n);
end
% 记录每一代的最优路径长度
bestlen = zeros(1,maxgen);
% 迭代遗传
for gen = 1:maxgen
% 计算适应度
fit = zeros(1,popsize);
for i = 1:popsize
fit(i) = fitness(pop(i,:),D);
end
% 记录最优路径长度
bestlen(gen) = min(fit);
% 选择
newpop = zeros(popsize,n);
for i = 1:popsize
% 轮盘赌选择
idx1 = randi(popsize);
idx2 = randi(popsize);
if fit(idx1) < fit(idx2)
parent1 = pop(idx1,:);
else
parent1 = pop(idx2,:);
end
idx1 = randi(popsize);
idx2 = randi(popsize);
if fit(idx1) < fit(idx2)
parent2 = pop(idx1,:);
else
parent2 = pop(idx2,:);
end
% 交叉
if rand < pc
child = crossover(parent1,parent2);
else
child = parent1;
end
% 变异
if rand < pm
child = mutation(child);
end
newpop(i,:) = child;
end
% 更新种群
pop = newpop;
% 绘制当前最优路径
bestidx = find(fit == min(fit),1);
bestpath = pop(bestidx,:);
plot3(C(bestpath,1),C(bestpath,2),C(bestpath,3),'LineWidth',2);
hold on;
end
% 绘制城市坐标
scatter3(C(:,1),C(:,2),C(:,3),'filled');
% 输出最优路径长度
fprintf('最优路径长度: %f\n',bestlen(end));
function f = fitness(path,D)
% 计算路径的适应度
f = 1/totaldistance(path,D);
end
function d = totaldistance(path,D)
% 计算路径的总距离
n = length(path);
d = 0;
for i = 1:n-1
d = d + D(path(i),path(i+1));
end
d = d + D(path(n),path(1));
end
function child = crossover(parent1,parent2)
% 交叉操作
n = length(parent1);
child = zeros(1,n);
start = randi(n);
stop = randi(n);
if start > stop
temp = start;
start = stop;
stop = temp;
end
child(start:stop) = parent1(start:stop);
for i = 1:n
if ~ismember(parent2(i),child)
for j = 1:n
if child(j) == 0
child(j) = parent2(i);
break;
end
end
end
end
end
function child = mutation(parent)
% 变异操作
n = length(parent);
idx1 = randi(n);
idx2 = randi(n);
temp = parent(idx1);
parent(idx1) = parent(idx2);
parent(idx2) = temp;
child = parent;
end
```
在绘制最优路径时,我使用了MATLAB的3D绘图函数`plot3`,将城市坐标的x、y、z分别传入,绘制出三维路径。最后输出了最优路径长度。
阅读全文
相关推荐





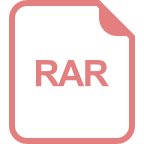
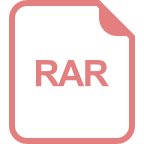






