用python torch:使用iris.csv数据构建神经网络预测花的种类:将所有数据随机分为训练集和测试集,分别计算测试集上总的准确率和不同类的准确率。
时间: 2024-02-11 11:08:34 浏览: 85
好的,首先需要导入必要的库和数据集:
```python
import torch
import torch.nn as nn
import pandas as pd
from sklearn.model_selection import train_test_split
# 读取数据集
iris = pd.read_csv('iris.csv')
# 将类别转换为数字
iris['Species'] = pd.Categorical(iris['Species'])
iris['Species'] = iris['Species'].cat.codes
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(iris.iloc[:,:-1].values, iris.iloc[:,-1].values, test_size=0.2, random_state=42)
```
接下来,我们需要定义一个三层的神经网络模型:
```python
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(4, 16)
self.fc2 = nn.Linear(16, 8)
self.fc3 = nn.Linear(8, 3)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.relu(self.fc2(x))
x = self.fc3(x)
return x
```
接着,我们需要定义一些训练参数:
```python
net = Net()
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(net.parameters(), lr=0.01)
```
然后,我们可以开始训练模型:
```python
# 设置训练轮数
epochs = 1000
for epoch in range(epochs):
optimizer.zero_grad()
inputs = torch.tensor(X_train, dtype=torch.float32)
labels = torch.tensor(y_train, dtype=torch.long)
outputs = net(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
# 计算训练集上的准确率
with torch.no_grad():
inputs = torch.tensor(X_train, dtype=torch.float32)
labels = torch.tensor(y_train, dtype=torch.long)
outputs = net(inputs)
_, predicted = torch.max(outputs.data, 1)
correct = (predicted == labels).sum().item()
train_acc = correct / len(y_train)
# 计算测试集上的准确率
with torch.no_grad():
inputs = torch.tensor(X_test, dtype=torch.float32)
labels = torch.tensor(y_test, dtype=torch.long)
outputs = net(inputs)
_, predicted = torch.max(outputs.data, 1)
correct = (predicted == labels).sum().item()
test_acc = correct / len(y_test)
# 输出训练进度
if (epoch+1) % 100 == 0:
print('Epoch [{}/{}], Loss: {:.4f}, Train Acc: {:.2f}%, Test Acc: {:.2f}%'
.format(epoch+1, epochs, loss.item(), train_acc*100, test_acc*100))
```
最后,我们可以输出不同类别的准确率:
```python
with torch.no_grad():
inputs = torch.tensor(X_test, dtype=torch.float32)
labels = torch.tensor(y_test, dtype=torch.long)
outputs = net(inputs)
_, predicted = torch.max(outputs.data, 1)
for i in range(3):
indices = (labels == i)
class_acc = (predicted[indices] == labels[indices]).float().mean()
print('Accuracy of class %d: %.2f%%' % (i, class_acc*100))
```
完整代码如下:
阅读全文
相关推荐
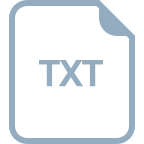
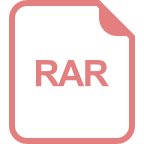






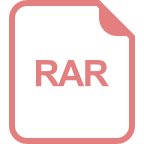
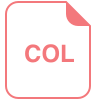
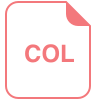
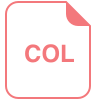
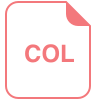
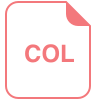
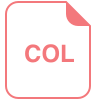
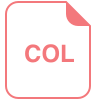
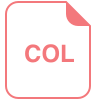
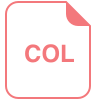