利用C语言编写代码,描述位错在特定滑移系上面运动
时间: 2023-09-19 09:06:47 浏览: 189
### 回答1:
可以使用C语言编写代码来描述位错在特定滑移系上的运动。
首先,需要定义滑移系的结构,并将位错的初始位置记录在其中。
然后,根据特定的运动模型,模拟位错在滑移系上的运动。例如,可以使用随机游走模型来模拟位错的随机移动。
在每一步运动中,需要更新位错的位置,并使用相关的算法计算出下一步的运动方向。
最后,根据模拟的结果,可以得出位错在滑移系上的运动轨迹以及其它相关信息。
代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define N 10 //滑移系的大小
#define T 100 //模拟的步数
int main() {
int i, j, k;
int lattice[N][N][N]; //定义滑移系
int x, y, z; //定义位错的位置
int dx, dy, dz; //定义位错的移动方向
srand(time(NULL)); //初始化随机数生成器
//初始化滑移系和位错的位置
for (i = 0; i < N; i++) {
for (j = 0; j < N; j++) {
for (k = 0; k < N; k++) {
lattice[i][j][k] = 0;
}
}
}
x = N / 2;
y = N / 2;
z = N / 2;
lattice[x][y][z] = 1;
//模拟位错的运动
for (
### 回答2:
位错是晶体中晶格畸变的一种现象,可以通过编写C语言代码来描述其在特定滑移系上的运动。
首先,我们需要定义晶格的基本信息,如晶胞参数和晶格坐标。可以使用结构体定义晶胞:
```
typedef struct {
double a, b, c; // 晶胞参数
double alpha, beta, gamma; // 晶胞角度
} Lattice;
typedef struct {
double x, y, z; // 原子的坐标
} Atom;
```
接下来,我们可以定义位错的结构体和相关函数:
```
typedef struct {
Atom position; // 位错的位置
Atom burgers; // 位错的Burgers矢量
} Dislocation;
void slip(Dislocation *dislocation, Lattice *lattice) {
// 根据滑移位移和晶胞参数计算滑移位错的新位置
dislocation->position.x += lattice->a * slipParameter;
dislocation->position.y += lattice->b * slipParameter;
dislocation->position.z += lattice->c * slipParameter;
}
```
通过上述代码,我们可以使位错在特定滑移系上运动。在主函数中,可以进行位错的初始化,并调用`slip`函数进行滑移。
```
int main() {
Lattice lattice;
Dislocation dislocation;
// 初始化晶胞和位错的信息
lattice.a = 5.0;
lattice.b = 5.0;
lattice.c = 10.0;
lattice.alpha = 90;
lattice.beta = 90;
lattice.gamma = 120;
dislocation.position.x = 0.0;
dislocation.position.y = 0.0;
dislocation.position.z = 0.0;
dislocation.burgers.x = 1.0;
dislocation.burgers.y = -1.0;
dislocation.burgers.z = 0.0;
// 进行滑移
slip(&dislocation, &lattice);
return 0;
}
```
以上代码只是简单地展示了如何使用C语言描述位错在特定滑移系上的运动,实际情况需要根据具体滑移系和位错类型进行适当的修改。
### 回答3:
位错是固态材料中晶体结构中的一种缺陷,可以通过C语言编写代码来描述位错在特定滑移系上面的运动。
在C语言中,可以定义一个位错结构体,用来存储位错的位置、类型等信息。例如:
```
typedef struct {
int x; // 位错的x坐标
int y; // 位错的y坐标
int type; // 位错的类型
} Dislocation;
```
然后,我们可以编写一个函数来模拟位错的运动。这个函数接收一个位错结构体作为参数,根据位错类型和特定滑移系的方向,对位错的位置进行更新。例如:
```
void dislocationMotion(Dislocation* dislocation) {
// 根据位错类型和特定滑移系的方向,更新位错的位置
// 这里假设位错类型为1,滑移方向为正x方向
if (dislocation->type == 1) {
dislocation->x += 1;
}
}
```
在主函数中,我们可以创建一个位错结构体实例,调用位错运动函数,然后打印出位错的位置信息。例如:
```
int main() {
Dislocation dislocation = {0, 0, 1}; // 创建位错结构体实例,初始位置为(0, 0),类型为1
dislocationMotion(&dislocation); // 调用位错运动函数,更新位错位置
printf("位错位置:(%d, %d)\n", dislocation.x, dislocation.y); // 打印位错位置信息
return 0;
}
```
通过以上代码,我们可以描述位错在特定滑移系上面的运动。根据位错的类型和滑移方向,可以灵活地更新位错的位置,模拟位错在材料中的运动行为。
阅读全文
相关推荐
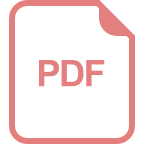



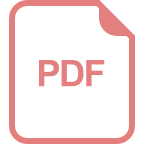
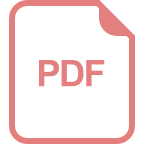
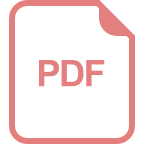
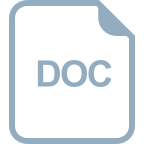







