设计一个学生类,包含学生学号、姓名和成绩。计算3名学生的平均成绩。 高级要求:利用运算符重载的方式计算总成绩; 高级要求:将学生类单独打包成模块。
时间: 2024-12-15 15:20:02 浏览: 10
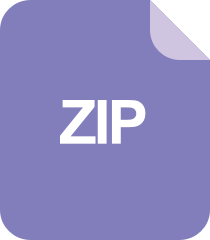
一个基于 C++ 编写的学生成绩管理系统 + 源代码 + 详细文档
首先,我们可以设计一个名为`Student`的类,包含基本属性如学号、姓名和成绩:
```java
public class Student {
private int studentId;
private String name;
private double score;
// 构造函数
public Student(int id, String name, double score) {
this.studentId = id;
this.name = name;
this.score = score;
}
// 访问器方法
public int getStudentId() { return studentId; }
public String getName() { return name; }
public double getScore() { return score; }
// 运算符重载 - 总分加法
public static double addScore(Student... students) {
double total = 0;
for (Student s : students) {
total += s.getScore();
}
return total;
}
}
```
接下来,为了计算三名学生的平均成绩,我们可以在主程序中创建三个`Student`对象并调用`addScore`方法,然后除以学生数:
```java
public class Main {
public static void main(String[] args) {
Student student1 = new Student(1, "Alice", 85);
Student student2 = new Student(2, "Bob", 90);
Student student3 = new Student(3, "Charlie", 95);
double averageScore = Student.addScore(student1, student2, student3) / 3;
System.out.println("The average score of the three students is: " + averageScore);
}
}
```
对于高级要求,我们将`Student`类放在一个单独的模块(通常是一个`.java`文件或一个包中),例如`edu.example.students`。然后在其他需要使用这个类的地方导入它:
```java
// 引入学生模块
import edu.example.students.Student;
public class Main {
//...
}
```
阅读全文
相关推荐
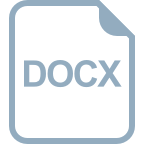
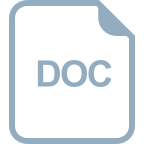





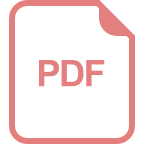
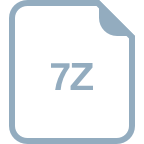
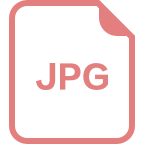
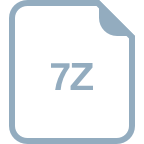
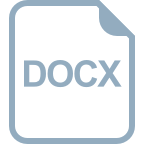