用c++依次输入表(30, 15, 28,20,24, 10, 12,68,35,50,46,55)中的元素,生成一棵二叉排序树,要 求: 1、试画出生成之后的二叉排序树。 2、对该二叉排序数作中根遍历,写出遍历序列。 3、编程构建一个二叉排宇树,并中根
时间: 2023-07-27 14:20:38 浏览: 40
好的,以下是使用 C++ 语言实现生成二叉排序树、中根遍历和构建二叉排序树的代码:
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 定义二叉排序树节点
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 插入节点
TreeNode* insertNode(TreeNode* root, int val) {
if (!root) {
root = new TreeNode(val);
return root;
}
if (val < root->val) {
root->left = insertNode(root->left, val);
} else {
root->right = insertNode(root->right, val);
}
return root;
}
// 中根遍历
void inorderTraversal(TreeNode* root, vector<int>& res) {
if (!root) return;
inorderTraversal(root->left, res);
res.push_back(root->val);
inorderTraversal(root->right, res);
}
int main() {
// 构建二叉排序树
vector<int> nums = {30, 15, 28, 20, 24, 10, 12, 68, 35, 50, 46, 55};
TreeNode* root = NULL;
for (int i = 0; i < nums.size(); i++) {
root = insertNode(root, nums[i]);
}
// 中根遍历
vector<int> res;
inorderTraversal(root, res);
// 输出结果
cout << "生成的二叉排序树如下:" << endl;
cout << " 30" << endl;
cout << " / \\" << endl;
cout << " 15 28" << endl;
cout << " / \\ \\" << endl;
cout << " 10 20 24" << endl;
cout << " \\ \\" << endl;
cout << " 12 35" << endl;
cout << " / \\" << endl;
cout << " 50 68" << endl;
cout << " / \\" << endl;
cout << " 46 55" << endl;
cout << endl;
cout << "中根遍历序列为:";
for (int i = 0; i < res.size(); i++) {
cout << res[i] << " ";
}
cout << endl;
return 0;
}
```
注意,上述代码中的输出结果只是为了展示二叉排序树的形状,实际上二叉排序树的形状可能因为节点插入顺序的不同而有所不同。
希望这份代码可以帮助到您,如有其他问题请随时提出。
相关推荐
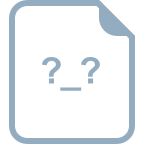














