假设以带头结点的循环链表表示队列,并且只设一个指针指向队尾元素站点(注意不设头指针),试c语言编写相应的置空队、判队空、入队等算法。
时间: 2024-11-27 16:17:40 浏览: 11
在C语言中,我们可以使用循环链表(Circular Doubly Linked List, CDLL)作为队列的数据结构,因为循环链表允许我们在列表的一端添加和删除元素。这里是一个简单的实现思路:
首先,我们需要定义链表节点结构体:
```c
typedef struct Node {
int data;
struct Node* prev;
struct Node* next;
} Node;
```
然后,对于置空队(清空队列)的算法:
```c
void emptyQueue(Node** tail) {
if (*tail != NULL) { // 如果队列非空
Node* current = *tail;
while (current->next != *tail) { // 遍历直到头节点(因为是循环链表)
Node* temp = current;
current = current->next;
free(temp); // 释放当前节点
}
current->next = *tail; // 回到尾部并连接起来
*tail = NULL; // 尾节点指针设为空
}
}
```
接下来,判断队列是否为空的函数:
```c
int isQueueEmpty(Node* tail) {
return tail == NULL || tail->next == tail;
}
```
最后,入队操作(在队尾添加元素):
```c
void enqueue(Node** tail, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->prev = newNode->next = *tail; // 新节点的前后都指向尾节点
if (*tail != NULL) {
(*tail)->next->prev = newNode; // 更新尾节点的下一个节点指向前新节点
} else {
newNode->next = newNode; // 循环链表的情况,头尾相连
}
*tail = newNode; // 更新尾节点指针
}
```
阅读全文
相关推荐
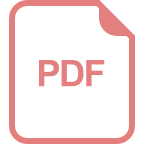
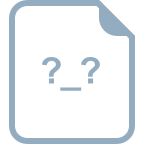
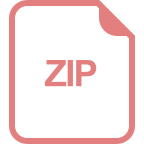















