假设以带头结点的循环链表表示队列,并且只设一个指针指向队尾元素结点(注意不设头指针),试编写相应的队列初始化、入队列和出队列的算法。c语言实现
时间: 2024-11-28 18:04:27 浏览: 6
当使用循环链表表示队列时,由于队列的特点(后进先出,LIFO),我们只需要两个操作:入队(enqueue)和出队(dequeue)。因为只有一个指针指向队尾,我们需要找到最后一个元素并更新这个指针。
首先,让我们定义一个结构体表示链表节点:
```c
typedef struct Node {
int data;
struct Node *next;
} Node;
```
然后,创建一个队列结构,包含一个指向当前队尾的指针:
```c
typedef struct Queue {
Node *tail;
} Queue;
```
**初始化队列(Queue_Init):**
- 初始化时,队列为空,`tail` 指针为 `NULL`。
```c
void Queue_Init(Queue *q) {
q->tail = NULL;
}
```
**入队操作(Enqueue):**
- 首先检查队列是否已满(对于循环链表,这里默认队列为无限大,实际上不需要特殊处理队满的情况)。
- 如果队尾非空,则新建一个节点,并将其`next`指向当前队尾,然后更新队尾指针。
```c
void Enqueue(Queue *q, int value) {
Node *new_node = (Node *)malloc(sizeof(Node));
if (new_node) {
new_node->data = value;
new_node->next = q->tail;
// 循环链表设置尾部链接到头部
if (q->tail == NULL) {
new_node->next = new_node;
} else {
q->tail->next = new_node;
}
q->tail = new_node;
} else {
printf("Memory allocation failed.\n");
}
}
```
**出队操作(Dequeue):**
- 如果队列为空,返回错误或抛出异常。
- 否则,删除当前队尾节点,返回其数据,并将`tail`指针移到下一个节点。
```c
int Dequeue(Queue *q) {
if (q->tail == NULL) {
return -1; // 或者抛出异常
}
int value = q->tail->data;
Node *temp = q->tail;
q->tail = q->tail->next; // 更新尾指针
free(temp); // 释放内存
return value;
}
```
以上就是使用循环链表表示队列的基本操作。队列的入队操作始终向尾部添加新元素,而出队操作总是移除尾部元素。
阅读全文
相关推荐
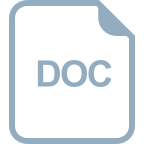
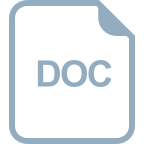
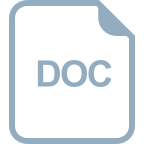










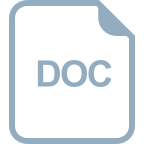
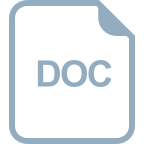
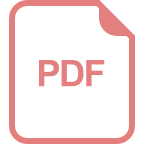
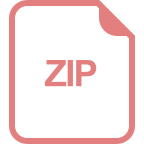
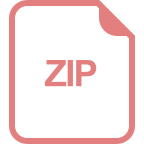