帮我写一份PWM的代码
时间: 2023-08-28 19:06:23 浏览: 106
当涉及到编写PWM(脉宽调制)代码时,具体的实现方式会根据使用的硬件平台和编程语言而有所不同。下面是一个使用C语言编写PWM代码的示例,假设你使用的是基于Linux的系统,并且有一个可用的GPIO(通用输入输出)引脚来生成PWM信号。
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#define PWM_CHIP_PATH "/sys/class/pwm/pwmchip0"
#define PWM_EXPORT_PATH "/sys/class/pwm/pwmchip0/export"
#define PWM_UNEXPORT_PATH "/sys/class/pwm/pwmchip0/unexport"
// 设置PWM周期和占空比
void setPWM(int period, int duty_cycle) {
// 打开PWM设备
int export_fd = open(PWM_EXPORT_PATH, O_WRONLY);
if (export_fd == -1) {
perror("无法打开PWM设备");
exit(1);
}
// 导出PWM通道
write(export_fd, "0", 1);
close(export_fd);
// 设置PWM周期
char period_path[100];
sprintf(period_path, "%s/pwm0/period", PWM_CHIP_PATH);
int period_fd = open(period_path, O_WRONLY);
if (period_fd == -1) {
perror("无法打开周期文件");
exit(1);
}
dprintf(period_fd, "%d", period);
close(period_fd);
// 设置PWM占空比
char duty_cycle_path[100];
sprintf(duty_cycle_path, "%s/pwm0/duty_cycle", PWM_CHIP_PATH);
int duty_cycle_fd = open(duty_cycle_path, O_WRONLY);
if (duty_cycle_fd == -1) {
perror("无法打开占空比文件");
exit(1);
}
dprintf(duty_cycle_fd, "%d", duty_cycle);
close(duty_cycle_fd);
// 启用PWM输出
char enable_path[100];
sprintf(enable_path, "%s/pwm0/enable", PWM_CHIP_PATH);
int enable_fd = open(enable_path, O_WRONLY);
if (enable_fd == -1) {
perror("无法打开使能文件");
exit(1);
}
write(enable_fd, "1", 1);
close(enable_fd);
}
// 停止PWM输出
void stopPWM() {
// 关闭PWM输出
char enable_path[100];
sprintf(enable_path, "%s/pwm0/enable", PWM_CHIP_PATH);
int enable_fd = open(enable_path, O_WRONLY);
if (enable_fd == -1) {
perror("无法打开使能文件");
exit(1);
}
write(enable_fd, "0", 1);
close(enable_fd);
// 取消导出PWM通道
int unexport_fd = open(PWM_UNEXPORT_PATH, O_WRONLY);
if (unexport_fd == -1) {
perror("无法打开取消导出文件");
exit(1);
}
write(unexport_fd, "0", 1);
close(unexport_fd);
}
int main() {
int period = 1000000; // PWM周期,单位为纳秒(1ms)
int duty_cycle = 200000; // PWM占空比,单位为纳秒(0.2ms)
setPWM(period, duty_cycle);
sleep(5); // 持续输出PWM信号5秒
stopPWM();
return 0;
}
```
请注意,上述代码仅供参考,并假设你的系统上存在适当的PWM设备和GPIO引脚。具体的路径和数值应根据你的系统进行调整。此外,这只是一个简单的示例,你可能需要根据自己的需求进行更多的功能扩展和错误处理。
阅读全文
相关推荐
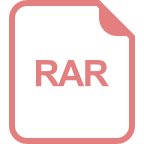














