用python生成一边录音一边显示FFT画布的多线程程序
时间: 2024-05-07 21:15:24 浏览: 40
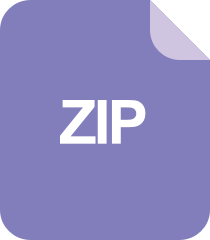
vc 同时录音和播放录音的多线程程序

以下是用Python编写一边录音一边显示FFT画布的多线程程序的示例代码:
```python
import threading
import pyaudio
import numpy as np
import matplotlib.pyplot as plt
# 设置录音参数
CHUNK_SIZE = 1024
FORMAT = pyaudio.paInt16
CHANNELS = 1
RATE = 44100
# 创建PyAudio对象
p = pyaudio.PyAudio()
# 创建FFT画布
fig, ax = plt.subplots()
x = np.arange(0, CHUNK_SIZE, 1)
line, = ax.plot(x, np.random.rand(CHUNK_SIZE), '-', lw=2)
ax.set_ylim(0, 255)
ax.set_xlim(0, CHUNK_SIZE)
plt.ion()
plt.show()
# 创建用于录音的线程
class Recorder(threading.Thread):
def __init__(self):
threading.Thread.__init__(self)
self.frames = []
def run(self):
stream = p.open(format=FORMAT,
channels=CHANNELS,
rate=RATE,
input=True,
frames_per_buffer=CHUNK_SIZE)
while True:
data = stream.read(CHUNK_SIZE)
self.frames.append(data)
def get_frames(self):
return self.frames
# 创建用于绘制FFT画布的线程
class Plotter(threading.Thread):
def __init__(self, recorder):
threading.Thread.__init__(self)
self.recorder = recorder
def run(self):
while True:
frames = self.recorder.get_frames()
if len(frames) > 0:
data = b''.join(frames)
y = np.frombuffer(data, dtype=np.int16)
yf = np.abs(np.fft.fft(y))/CHUNK_SIZE
line.set_ydata(yf)
fig.canvas.draw()
fig.canvas.flush_events()
# 启动录音和绘制线程
recorder = Recorder()
recorder.start()
plotter = Plotter(recorder)
plotter.start()
```
该程序创建了两个线程,一个用于录音,另一个用于绘制FFT画布。录音线程将音频数据存储在一个列表中,绘制线程从列表中获取音频数据并计算FFT,然后更新画布。程序使用PyAudio库来处理音频输入,使用matplotlib库来绘制FFT画布。在绘制线程中,通过plt.ion()和plt.show()来实现动态绘制画布。
阅读全文
相关推荐
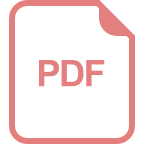
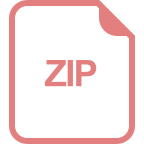








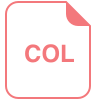
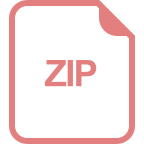
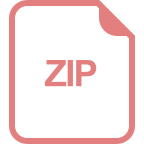
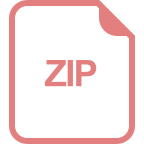
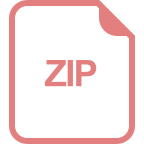