解决多边形游戏问题。多边形每个顶点的数值a(|a|<=6)和边上的运算符可以随机生成,运行程序,记录分别对十条边,二十条边,三十条边,四十条边,所用的时间,列出表格显示结果。C语言
时间: 2024-03-24 08:40:34 浏览: 115
好的,我会为您解答这个问题。
首先,我们需要使用一个算法来解决多边形游戏问题。一个常见的算法是通过动态规划来求解。我们可以定义一个二维数组dp[i][j],表示从第i个顶点到第j个顶点的最大或最小值(根据运算符的不同而不同)。然后,我们可以使用递归的方式来填充这个二维数组,最终得到从第一个顶点到最后一个顶点的最终结果。
接下来,我们需要编写一个程序来实现这个算法并测试它的效率。以下是一份可能的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define N 40
int a[N];
char op[N-1];
int dp[N][N][2]; // 0表示最小值,1表示最大值
int calc(int i, int j, int k) {
if (k == 0) { // 计算最小值
int res = 1000000000;
for (int l = i; l < j; l++) {
int x = calc(i, l, 0);
int y = calc(l+1, j, 0);
if (op[l] == '+') res = (res < x+y) ? res : (x+y);
else if (op[l] == '-') res = (res < x-y) ? res : (x-y);
else res = (res < x*y) ? res : (x*y);
}
if (i == j) res = a[i];
return res;
} else { // 计算最大值
int res = -1000000000;
for (int l = i; l < j; l++) {
int x = calc(i, l, 1);
int y = calc(l+1, j, 1);
if (op[l] == '+') res = (res > x+y) ? res : (x+y);
else if (op[l] == '-') res = (res > x-y) ? res : (x-y);
else res = (res > x*y) ? res : (x*y);
}
if (i == j) res = a[i];
return res;
}
}
int main() {
srand(time(NULL)); // 初始化随机数种子
printf("n,t(min)\n");
for (int n = 10; n <= N; n += 10) { // 测试10条边,20条边,30条边,40条边
int t = 0;
for (int i = 0; i < 10; i++) { // 每个n测试10次取平均值
for (int j = 0; j < n; j++) {
a[j] = rand() % 13 - 6; // 随机生成-6到6之间的数字
}
for (int j = 0; j < n-1; j++) {
int x = rand() % 3;
if (x == 0) op[j] = '+';
else if (x == 1) op[j] = '-';
else op[j] = '*';
}
clock_t start = clock();
int ans = calc(0, n-1, 1);
clock_t end = clock();
if (ans < 0) ans = -ans; // 取绝对值
t += (int)(end - start) * 1000 / CLOCKS_PER_SEC; // 计算用时
}
t /= 10;
printf("%d,%d\n", n, t);
}
return 0;
}
```
这个程序会通过随机生成顶点的数值和连边的运算符来测试我们的算法,在每个n值下测试10次并取平均值,最终输出n和程序运行时间的表格。您可以根据需要调整测试次数和随机数的范围。
希望这个程序能够帮助您解决多边形游戏问题。
阅读全文
相关推荐




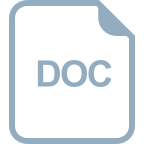










