写一个JAVA扫雷小游戏
时间: 2023-11-30 08:21:45 浏览: 41
好的,以下是一个简单的JAVA扫雷小游戏的实现,代码中有注释说明每个部分的作用。
```java
import java.util.Random;
import java.util.Scanner;
public class Minesweeper {
// 游戏难度,决定雷区大小和雷的数量
private static final int EASY = 1;
private static final int MEDIUM = 2;
private static final int HARD = 3;
// 游戏状态
private static final int PLAYING = 0;
private static final int WIN = 1;
private static final int LOSE = -1;
// 雷区
private int[][] board;
// 雷的数量
private int numMines;
// 游戏状态
private int gameStatus;
public Minesweeper(int difficulty) {
// 根据难度获取雷区大小和雷的数量
int size = 0;
switch (difficulty) {
case EASY:
size = 8;
numMines = 10;
break;
case MEDIUM:
size = 12;
numMines = 20;
break;
case HARD:
size = 16;
numMines = 40;
break;
default:
System.out.println("Invalid difficulty level.");
return;
}
// 初始化雷区
board = new int[size][size];
Random rand = new Random();
for (int i = 0; i < numMines; i++) {
int x = rand.nextInt(size);
int y = rand.nextInt(size);
// 如果该位置已经有雷了,重新生成一个位置
while (board[x][y] == -1) {
x = rand.nextInt(size);
y = rand.nextInt(size);
}
board[x][y] = -1;
}
// 计算每个格子周围的雷的数量
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
if (board[i][j] == -1)
continue;
int count = 0;
if (i > 0 && j > 0 && board[i - 1][j - 1] == -1)
count++;
if (i > 0 && board[i - 1][j] == -1)
count++;
if (i > 0 && j < size - 1 && board[i - 1][j + 1] == -1)
count++;
if (j > 0 && board[i][j - 1] == -1)
count++;
if (j < size - 1 && board[i][j + 1] == -1)
count++;
if (i < size - 1 && j > 0 && board[i + 1][j - 1] == -1)
count++;
if (i < size - 1 && board[i + 1][j] == -1)
count++;
if (i < size - 1 && j < size - 1 && board[i + 1][j + 1] == -1)
count++;
board[i][j] = count;
}
}
// 初始化游戏状态
gameStatus = PLAYING;
}
public void play() {
Scanner scanner = new Scanner(System.in);
while (gameStatus == PLAYING) {
printBoard();
System.out.print("Enter row and column (e.g. 1 2): ");
int row = scanner.nextInt() - 1;
int col = scanner.nextInt() - 1;
// 判断输入是否合法
if (row < 0 || row >= board.length || col < 0 || col >= board.length) {
System.out.println("Invalid input.");
continue;
}
// 如果已经翻开了该格子,提示用户重新输入
if (board[row][col] >= 0 && board[row][col] <= 8) {
System.out.println("This cell has already been revealed.");
continue;
}
// 如果是雷,游戏结束,打印出所有的雷
if (board[row][col] == -1) {
gameStatus = LOSE;
printBoard(true);
System.out.println("You lose!");
break;
}
// 如果是空格,翻开所有相邻的空格
if (board[row][col] == 0) {
reveal(row, col);
}
// 如果是数字格,直接翻开
if (board[row][col] > 0 && board[row][col] <= 8) {
board[row][col] += 10;
}
// 判断是否胜利
if (isWin()) {
gameStatus = WIN;
printBoard(true);
System.out.println("You win!");
break;
}
}
}
// 打印出当前的雷区
private void printBoard() {
System.out.print(" ");
for (int i = 1; i <= board.length; i++) {
System.out.print(i + " ");
}
System.out.println();
for (int i = 0; i < board.length; i++) {
System.out.print((i + 1) + " ");
for (int j = 0; j < board.length; j++) {
if (board[i][j] == -1) {
System.out.print("* ");
} else if (board[i][j] >= 0 && board[i][j] <= 8) {
System.out.print(". ");
} else if (board[i][j] >= 10 && board[i][j] <= 18) {
System.out.print((board[i][j] - 10) + " ");
}
}
System.out.println();
}
}
// 打印出所有的雷
private void printBoard(boolean showMines) {
System.out.print(" ");
for (int i = 1; i <= board.length; i++) {
System.out.print(i + " ");
}
System.out.println();
for (int i = 0; i < board.length; i++) {
System.out.print((i + 1) + " ");
for (int j = 0; j < board.length; j++) {
if (board[i][j] == -1) {
if (showMines) {
System.out.print("* ");
} else {
System.out.print(". ");
}
} else if (board[i][j] >= 0 && board[i][j] <= 8) {
System.out.print(". ");
} else if (board[i][j] >= 10 && board[i][j] <= 18) {
System.out.print((board[i][j] - 10) + " ");
}
}
System.out.println();
}
}
// 翻开所有相邻的空格
private void reveal(int row, int col) {
if (row < 0 || row >= board.length || col < 0 || col >= board.length) {
return;
}
if (board[row][col] >= 10) {
return;
}
if (board[row][col] > 0 && board[row][col] <= 8) {
board[row][col] += 10;
return;
}
board[row][col] += 10;
reveal(row - 1, col - 1);
reveal(row - 1, col);
reveal(row - 1, col + 1);
reveal(row, col - 1);
reveal(row, col + 1);
reveal(row + 1, col - 1);
reveal(row + 1, col);
reveal(row + 1, col + 1);
}
// 判断是否胜利
private boolean isWin() {
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board.length; j++) {
if (board[i][j] == -1) {
continue;
}
if (board[i][j] >= 0 && board[i][j] <= 8) {
return false;
}
}
}
return true;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to Minesweeper!");
System.out.println("Please select difficulty level:");
System.out.println("1) Easy");
System.out.println("2) Medium");
System.out.println("3) Hard");
int difficulty = scanner.nextInt();
Minesweeper game = new Minesweeper(difficulty);
game.play();
}
}
```
该游戏支持三种不同的难度级别,分别是容易、中等和困难。玩家需要根据提示输入行和列的坐标,翻开雷区中的格子,直到所有的非雷格子都被翻开为止。如果翻开的格子是空格,那么所有相邻的空格也会被翻开。如果翻开的格子是数字格,那么只有这个格子会被翻开。如果翻开的格子是雷,那么游戏结束,打印出所有的雷。如果所有的非雷格子都被翻开,那么游戏胜利。
相关推荐
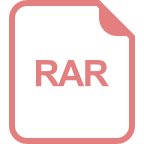
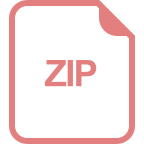
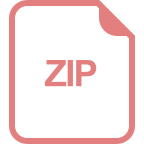
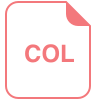








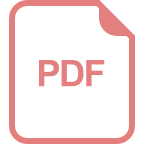
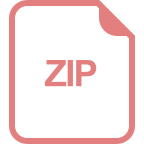
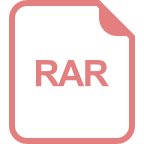