用c语言栈写一个二进制转换十进制的程序
时间: 2023-09-26 12:09:26 浏览: 45
好的,以下是一个用 C 语言栈实现二进制转换为十进制的程序:
```c
#include <stdio.h>
#define MAX_SIZE 100
// 栈的结构体定义
struct Stack {
int top;
int arr[MAX_SIZE];
};
// 初始化栈
void initStack(struct Stack *s) {
s->top = -1;
}
// 判断栈是否已满
int isFull(struct Stack *s) {
return s->top == MAX_SIZE - 1;
}
// 判断栈是否为空
int isEmpty(struct Stack *s) {
return s->top == -1;
}
// 入栈操作
void push(struct Stack *s, int item) {
if (isFull(s)) {
printf("Stack is full\n");
return;
}
s->arr[++s->top] = item;
}
// 出栈操作
int pop(struct Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty\n");
return -1;
}
return s->arr[s->top--];
}
// 二进制转换为十进制
int binaryToDecimal(char binary[]) {
struct Stack s;
initStack(&s);
int i = 0, decimal = 0, power = 1;
// 将二进制数的每一位依次入栈
while (binary[i] != '\0') {
push(&s, binary[i] - '0');
i++;
}
// 依次出栈并计算十进制数
while (!isEmpty(&s)) {
decimal += pop(&s) * power;
power *= 2;
}
return decimal;
}
int main() {
char binary[MAX_SIZE];
printf("Enter a binary number: ");
scanf("%s", binary);
int decimal = binaryToDecimal(binary);
printf("Decimal equivalent of %s is %d\n", binary, decimal);
return 0;
}
```
程序通过栈的数据结构来实现二进制转换为十进制,`push` 函数用来入栈,`pop` 函数用来出栈,`binaryToDecimal` 函数用来将二进制数转换为十进制数。在 `main` 函数中,读取用户输入的二进制数,并调用 `binaryToDecimal` 函数来计算十进制数的值,最后输出结果。
相关推荐
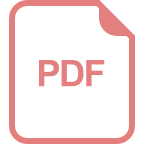
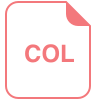
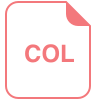
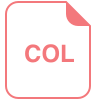
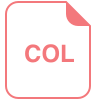
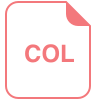









