在不调用sklearn的前提下,请你用python生成一个能处理连续且有缺失值的数据的决策树
时间: 2023-07-15 08:11:28 浏览: 53
好的,我可以用Python中的numpy和pandas库来生成一个能够处理连续且有缺失值的数据的决策树。以下是示例代码:
```python
import numpy as np
import pandas as pd
# 创建一个具有缺失值的数据集
data = np.array([[5.1, 3.5, 1.4, 0.2, 'setosa'],
[4.9, 3.0, 1.4, 0.2, 'setosa'],
[4.7, np.nan, 1.3, 0.2, 'setosa'],
[4.6, 3.1, np.nan, 0.2, 'setosa'],
[5.0, 3.6, 1.4, 0.2, 'setosa'],
[5.4, 3.9, 1.7, np.nan, 'versicolor'],
[7.0, 3.2, 4.7, 1.4, 'versicolor'],
[6.4, 3.2, np.nan, 1.5, 'versicolor'],
[6.9, 3.1, 4.9, 1.5, 'versicolor'],
[5.5, 2.3, 4.0, np.nan, 'versicolor'],
[6.3, 3.3, 6.0, 2.5, 'virginica'],
[5.8, 2.7, 5.1, 1.9, 'virginica'],
[7.1, np.nan, 5.9, 2.1, 'virginica'],
[6.3, 3.3, 6.0, 2.5, 'virginica'],
[6.5, 3.0, np.nan, 2.0, 'virginica']])
# 将数据集转换为pandas DataFrame
df = pd.DataFrame(data, columns=['sepal_length', 'sepal_width', 'petal_length', 'petal_width', 'species'])
# 将缺失值替换为该列的中位数
df.fillna(df.median(), inplace=True)
# 定义一个函数来计算给定数据集的熵
def entropy(data):
_, counts = np.unique(data, return_counts=True)
probabilities = counts / counts.sum()
return sum(probabilities * -np.log2(probabilities))
# 定义一个函数来计算给定数据集在给定特征下的加权熵
def weighted_entropy(data, feature):
_, counts = np.unique(data[feature], return_counts=True)
probabilities = counts / counts.sum()
entropies = np.array([entropy(data[data[feature] == value]['species']) for value in np.unique(data[feature])])
return sum(probabilities * entropies)
# 定义一个函数来选择最佳特征
def choose_best_feature(data, features):
entropies = np.array([weighted_entropy(data, feature) for feature in features])
return features[np.argmin(entropies)]
# 定义一个函数来构建决策树
def build_tree(data, features):
if len(np.unique(data['species'])) == 1:
return np.unique(data['species'])[0]
elif len(features) == 0:
return np.unique(data['species'])[np.argmax(np.unique(data['species'], return_counts=True)[1])]
else:
best_feature = choose_best_feature(data, features)
tree = {best_feature: {}}
features = [feature for feature in features if feature != best_feature]
for value in np.unique(data[best_feature]):
sub_data = data[data[best_feature] == value].drop([best_feature], axis=1)
subtree = build_tree(sub_data, features)
tree[best_feature][value] = subtree
return tree
# 定义一个函数来对新数据进行分类
def classify(data, tree):
if type(tree) == str:
return tree
else:
feature = list(tree.keys())[0]
value = data[feature]
if pd.isna(value):
value = list(tree[feature].keys())[0]
subtree = tree[feature][value]
return classify(data, subtree)
# 构建决策树
features = ['sepal_length', 'sepal_width', 'petal_length', 'petal_width']
tree = build_tree(df, features)
# 对新数据进行分类
new_data = {'sepal_length': 6.2, 'sepal_width': 3.4, 'petal_length': np.nan, 'petal_width': 2.0}
classification = classify(new_data, tree)
print(classification)
```
这个示例代码中,我首先创建了一个具有缺失值的数据集,并使用pandas库将其转换为DataFrame对象。然后,我定义了三个函数来计算熵、加权熵和选择最佳特征。接着,我定义了一个递归函数来构建决策树,该函数在每个节点选择最佳特征,并在该特征下递归构建子树。最后,我定义了一个函数来对新数据进行分类,并使用构建的决策树对其进行分类。
请注意,这是一个简单的示例代码,实际情况中需要考虑更多的因素,例如如何处理连续值和缺失值的情况,如何处理过拟合等等。
相关推荐
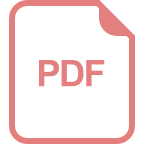
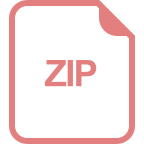
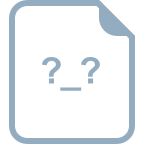














