写一个贪吃蛇的小游戏程序,用c语言
时间: 2023-09-27 21:05:36 浏览: 117
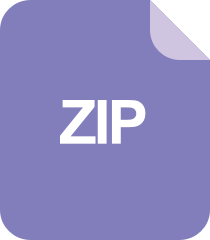
C语言期末大作业-C语言小游戏28个

下面是一个简单的贪吃蛇小游戏程序,使用C语言编写:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 30
#define HEIGHT 20
#define SNAKE_MAX_LENGTH (WIDTH * HEIGHT)
typedef struct _Point {
int x;
int y;
} Point;
typedef struct _Snake {
int length;
Point body[SNAKE_MAX_LENGTH];
} Snake;
typedef enum _Direction {
UP,
DOWN,
LEFT,
RIGHT
} Direction;
Point food;
Snake snake;
Direction direction = RIGHT;
void gotoxy(int x, int y) {
COORD pos = { x, y };
HANDLE hOut = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleCursorPosition(hOut, pos);
}
void initGame() {
srand((unsigned)time(NULL));
// 初始化蛇
snake.body[0] = (Point) { 10, 10 };
snake.body[1] = (Point) { 9, 10 };
snake.body[2] = (Point) { 8, 10 };
snake.length = 3;
// 初始化食物
food.x = rand() % WIDTH;
food.y = rand() % HEIGHT;
}
void draw() {
system("cls");
for (int i = 0; i < WIDTH + 2; i++) {
printf("#");
}
printf("\n");
for (int y = 0; y < HEIGHT; y++) {
printf("#");
for (int x = 0; x < WIDTH; x++) {
if (x == snake.body[0].x && y == snake.body[0].y) {
printf("O");
}
else if (x == food.x && y == food.y) {
printf("*");
}
else {
int isBody = 0;
for (int i = 1; i < snake.length; i++) {
if (x == snake.body[i].x && y == snake.body[i].y) {
printf("o");
isBody = 1;
break;
}
}
if (!isBody) {
printf(" ");
}
}
}
printf("#\n");
}
for (int i = 0; i < WIDTH + 2; i++) {
printf("#");
}
printf("\n");
}
void update() {
// 判断是否吃到食物
if (snake.body[0].x == food.x && snake.body[0].y == food.y) {
for (int i = snake.length; i > 0; i--) {
snake.body[i] = snake.body[i - 1];
}
snake.length++;
food.x = rand() % WIDTH;
food.y = rand() % HEIGHT;
}
// 更新蛇的位置
for (int i = snake.length - 1; i > 0; i--) {
snake.body[i] = snake.body[i - 1];
}
switch (direction) {
case UP:
snake.body[0].y--;
break;
case DOWN:
snake.body[0].y++;
break;
case LEFT:
snake.body[0].x--;
break;
case RIGHT:
snake.body[0].x++;
break;
}
// 判断是否碰到墙
if (snake.body[0].x < 0 || snake.body[0].x >= WIDTH || snake.body[0].y < 0 || snake.body[0].y >= HEIGHT) {
printf("Game Over!\n");
exit(0);
}
// 判断是否碰到自己的身体
for (int i = 1; i < snake.length; i++) {
if (snake.body[0].x == snake.body[i].x && snake.body[0].y == snake.body[i].y) {
printf("Game Over!\n");
exit(0);
}
}
}
void input() {
if (_kbhit()) {
switch (_getch()) {
case 'w':
case 'W':
if (direction != DOWN) {
direction = UP;
}
break;
case 's':
case 'S':
if (direction != UP) {
direction = DOWN;
}
break;
case 'a':
case 'A':
if (direction != RIGHT) {
direction = LEFT;
}
break;
case 'd':
case 'D':
if (direction != LEFT) {
direction = RIGHT;
}
break;
default:
break;
}
}
}
int main() {
initGame();
while (1) {
draw();
update();
input();
Sleep(100);
}
return 0;
}
```
该程序使用了Windows API的一些函数,因此只能在Windows平台下运行。在Windows下,可以使用Visual Studio等集成开发环境编译运行。
阅读全文
相关推荐
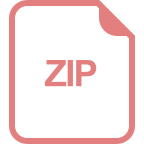
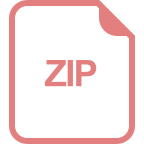













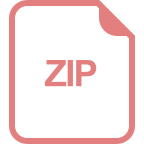
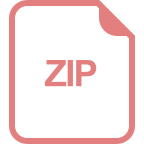
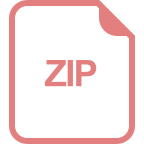