怎么用try catch来处理这段代码的异常:int i = licensePlateNumber.length() - 1; while (i >= 0 && !Character.isDigit(licensePlateNumber.charAt(i))) { i--; }
时间: 2023-11-28 14:51:10 浏览: 130
你可以使用以下代码块来使用 try-catch 来处理异常:
```
try {
int i = licensePlateNumber.length() - 1;
while (i >= 0 && !Character.isDigit(licensePlateNumber.charAt(i))) {
i--;
}
} catch (IndexOutOfBoundsException e) {
// 处理 IndexOutOfBoundsException 异常
e.printStackTrace();
} catch (Exception e) {
// 处理其他类型的异常
e.printStackTrace();
}
```
在上面的代码中,我们使用了两个 catch 块来捕获不同类型的异常。第一个 catch 块捕获 IndexOutOfBoundsException 异常,它将在试图访问具有无效索引的字符时抛出。第二个 catch 块捕获所有其他类型的异常。在 catch 块中,你可以编写适当的代码来处理异常。在这里,我们只是打印了异常堆栈跟踪。
相关问题
class ImageDownloader (private val url: String, private val imageView: ImageView, private val progressBar: ProgressBar) : Handler.Callback { private val handler = Handler(Looper.getMainLooper(), this) override fun handleMessage(msg: Message): Boolean { if (msg.what == 0) { imageView.setImageBitmap(msg.obj as Bitmap) progressBar.visibility = View.GONE } else if (msg.what == 1) { progressBar.progress = msg.arg1 } return true } fun download() { Thread { val bitmap = downloadImageFromUrl(url) handler.sendMessage(handler.obtainMessage(0, bitmap)) }.start() } private fun downloadImageFromUrl(url: String): Bitmap? { var bitmap: Bitmap? = null try { val urlConnection = URL(url).openConnection() urlConnection.connect() val inputStream = urlConnection.inputStream val totalSize = urlConnection.contentLength val data = ByteArray(1024) var downloadedSize = 0 var bufferLength: Int while (inputStream.read(data).also { bufferLength = it } != -1) { downloadedSize += bufferLength val progress = (downloadedSize * 100 / totalSize) handler.sendMessage(handler.obtainMessage(1, progress, 0)) bitmap = BitmapFactory.decodeByteArray(data, 0, bufferLength) } inputStream.close() } catch (e: Exception) { Log.e("ImageDownloader", "Error downloading image", e) } return bitmap } } 报出Process: com.example.imagedownloader, PID: 4842 java.lang.NullPointerException: null cannot be cast to non-null type android.graphics.Bitmap错误 修改代码
在 `downloadImageFromUrl` 函数中,应该将 `BitmapFactory.decodeByteArray` 转换成可空类型,以避免在无法解码字节数组时抛出 `NullPointerException`。修改后的代码如下:
```
private fun downloadImageFromUrl(url: String): Bitmap? {
var bitmap: Bitmap? = null
try {
val urlConnection = URL(url).openConnection()
urlConnection.connect()
val inputStream = urlConnection.inputStream
val totalSize = urlConnection.contentLength
val data = ByteArray(1024)
var downloadedSize = 0
var bufferLength: Int
while (inputStream.read(data).also { bufferLength = it } != -1) {
downloadedSize += bufferLength
val progress = (downloadedSize * 100 / totalSize)
handler.sendMessage(handler.obtainMessage(1, progress, 0))
bitmap = BitmapFactory.decodeByteArray(data, 0, bufferLength)?.let { it } // 加上安全转换
}
inputStream.close()
} catch (e: Exception) {
Log.e("ImageDownloader", "Error downloading image", e)
}
return bitmap
}
```
public void start(){ try { ClientRunnable clientRunnable = new ClientRunnable(); Thread thread = new Thread(clientRunnable); thread.setDaemon(true); thread.start(); OutputStream out = socket.getOutputStream(); OutputStreamWriter osw = new OutputStreamWriter(out, "utf-8"); BufferedWriter bw = new BufferedWriter(osw); PrintWriter pw = new PrintWriter(bw, true); Scanner input = new Scanner(System.in); while (true){ String line =input.nextLine(); String[] str = line.split("\\ +"); if(">>".equalsIgnoreCase(str[0])){ String ss=""; for (int i = 2; i < str.length; i++) { ss+=str[i]; if (i!=str.length-1) { ss+=" "; } } System.out.println("私聊" +str[1]+":"+ss); } if("exit".equalsIgnoreCase(line))break; pw.println(line); } } catch (IOException e) { e.printStackTrace(); } finally { try { socket.close(); } catch (IOException e) { e.printStackTrace(); } } }
这段代码是一个多线程聊天室客户端的start()方法。首先,创建一个ClientRunnable对象和一个Thread对象,将ClientRunnable对象作为参数传入Thread构造函数中。设置Thread为守护线程,并启动线程。接着,通过socket.getOutputStream()获取输出流,通过OutputStreamWriter将字符流转化为字节流,并指定字符编码为"utf-8"。再通过BufferedWriter对字节流进行缓冲写入。最后,通过PrintWriter将字符流转换为字节流,并自动刷新缓冲区,向服务器发送消息。使用Scanner读取用户在控制台输入的字符串,对输入的字符串进行判断,如果是私聊,则将私聊信息输出到控制台;如果是exit,则跳出循环,关闭客户端的socket连接;否则,将输入的字符串通过输出流发送给服务器。在finally块中,关闭socket连接。
阅读全文
相关推荐
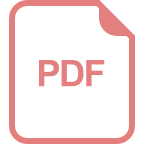
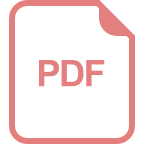














