java创建一个学生类(包括姓名,年龄,班级,密码),创建若干该类对象并保存在文件中(密码不保存),从文件读取对象后显示在屏幕上
时间: 2024-03-07 17:48:37 浏览: 36
好的,以下是一个示例代码,你可以参考它来完成你的任务:
```java
import java.io.*;
public class Student implements Serializable {
private String name;
private int age;
private String clazz;
private transient String password;
public Student(String name, int age, String clazz, String password) {
this.name = name;
this.age = age;
this.clazz = clazz;
this.password = password;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getClazz() {
return clazz;
}
public String getPassword() {
return password;
}
public String toString() {
return "Name: " + name + ", Age: " + age + ", Clazz: " + clazz;
}
public static void main(String[] args) {
Student[] students = {
new Student("Tom", 18, "Grade 1", "123456"),
new Student("Jerry", 19, "Grade 2", "abcdef"),
new Student("Lucy", 17, "Grade 3", "qwerty")
};
String filename = "students.dat";
// 存储学生对象到文件
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(filename))) {
for (Student student : students) {
oos.writeObject(student);
}
} catch (IOException e) {
e.printStackTrace();
}
// 从文件中读取学生对象并显示
try (ObjectInputStream ois = new ObjectInputStream(new FileInputStream(filename))) {
while (true) {
Student student = (Student) ois.readObject();
System.out.println(student);
}
} catch (EOFException e) {
// 文件读取结束
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
```
在上面的代码中,我们首先定义了一个Student类,包含姓名、年龄、班级和密码四个属性。其中,密码属性使用transient关键字修饰,表示该属性不需要被序列化保存。
接着,在main方法中,我们创建了三个学生对象,并将它们存储在一个数组中。然后,我们将学生对象序列化保存到文件students.dat中,使用ObjectOutputStream实现对象的序列化。
最后,我们使用ObjectInputStream从文件中读取序列化的学生对象,并将它们显示在屏幕上。注意,我们使用EOFException异常来判断文件是否读取结束。
希望这个示例能够帮助你完成你的任务。如果你有任何问题,可以继续向我提问。
相关推荐
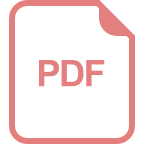











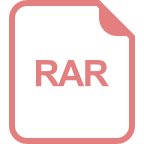
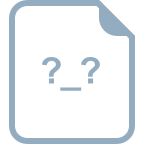
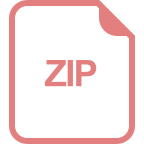