计算DQN模型准确率的python 代码
时间: 2023-12-25 08:45:43 浏览: 25
以下是一个简单的计算DQN模型准确率的Python代码示例:
```python
# 导入相关库
import gym
import numpy as np
import torch
from collections import deque
# 定义DQN模型
class DQN(torch.nn.Module):
def __init__(self):
super(DQN, self).__init__()
self.fc1 = torch.nn.Linear(4, 64)
self.fc2 = torch.nn.Linear(64, 2)
def forward(self, x):
x = torch.nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
# 初始化环境和模型
env = gym.make('CartPole-v1')
model = DQN()
model.load_state_dict(torch.load('dqn_model.pth'))
# 定义测试函数
def test(model):
scores = deque(maxlen=100)
for i in range(100):
state = env.reset()
done = False
score = 0
while not done:
action = np.argmax(model(torch.tensor(state, dtype=torch.float32)).detach().numpy())
state, reward, done, _ = env.step(action)
score += reward
scores.append(score)
return np.mean(scores)
# 计算准确率
accuracy = test(model)
print('Accuracy: {}'.format(accuracy))
```
该代码首先定义了一个简单的DQN模型,然后加载了训练好的模型权重,并定义了一个测试函数来计算模型在CartPole-v1环境下的平均得分。最后,计算并输出模型的准确率。
相关推荐
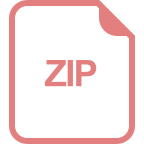
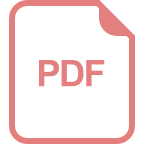








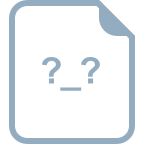
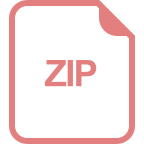
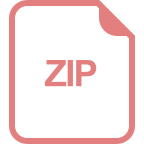